Automate News Collection with Google Apps Script: Fetch Daily Updates from Google News to Google Sheets
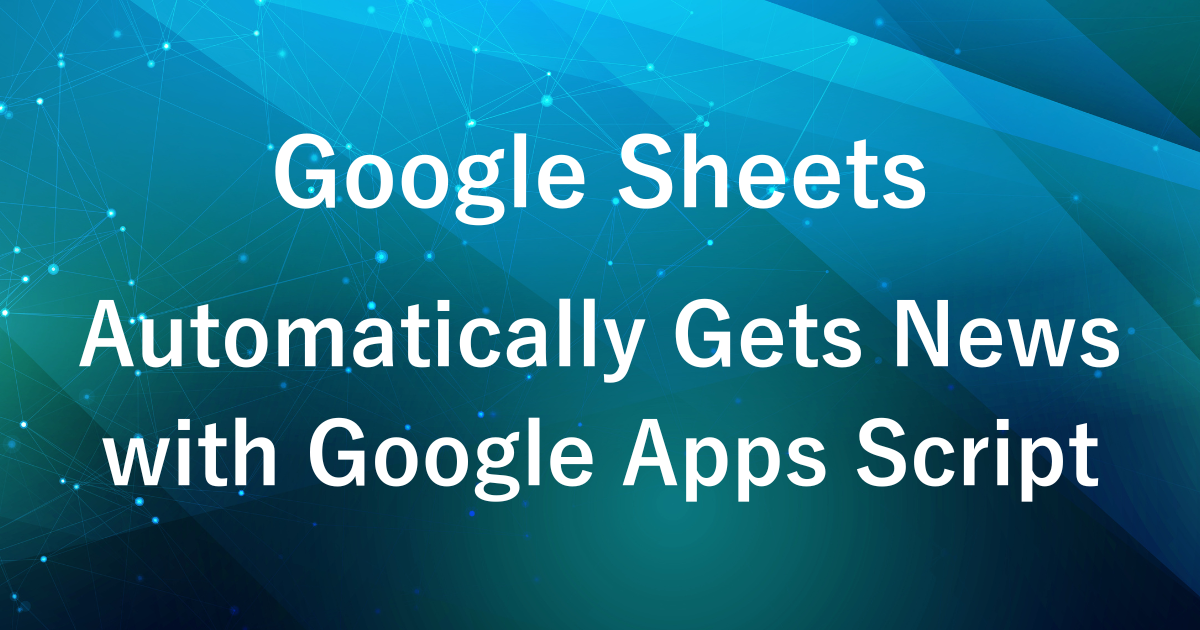
Do you want to organize your daily news information but find it time consuming to gather information?
It is also time-consuming to manually visit news sites.
So, in this article, we will show you how to use Google Apps Script to get Google News information into a spreadsheet.
Google News is a collection of articles from a variety of news sites, allowing for a broad collection of information.
This script allows you to easily manage your news without having to check multiple news sites.
You can retrieve by country or category, or filter by specific keywords.
Completed image
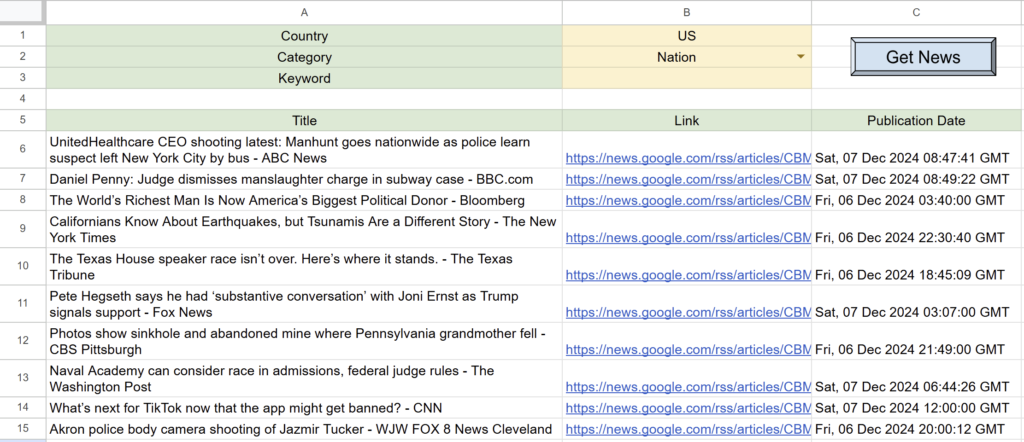
Retrieved from Google News
Column A: News title
Column B: Link
Column C: Publication date
Specify the country, category, and keywords in the spreadsheet, and news information retrieved using Google News RSS will be displayed.
About Google News RSS
Google News RSS is a feature that allows you to retrieve the latest news articles provided by Google News in feed format, by specific topic or country.
The feed format is a system that automatically distributes updates to news sites, blogs, and other websites when they are updated.
This allows you to efficiently receive new articles without having to manually visit each news site.
RSS (Really Simple Syndication) is an example of this feed format, which can be used to receive news of interest in bulk or linked to other tools such as spreadsheets to make information more manageable.
Procedure
Preparing Spreadsheets
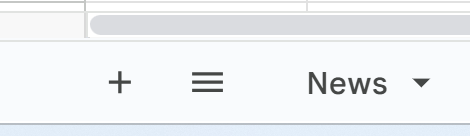
Set the sheet name to “News”.
The name of this sheet is referenced when the script is executed.

Enter the following field names in the spreadsheet
Field Name | |
---|---|
A1 | Country |
A2 | Category |
A3 | Keyword |
A5 | Title |
B5 | Link |
C5 | Publication Date |

Next, enter the news conditions you wish to retrieve.
Condition Input | |
---|---|
B1 | Country code such as US, JP, etc. |
B2 | Business, Technology, etc. |
B3 | Specific Keyword |
If no keywords are specified, news is retrieved by country and category only.
The countries and categories that can be retrieved with this script are as follows
Countries and categories that can be specified
- US (United States)
- JP (Japan)
- FR (France)
- DE (Germany)
- ES (Spain)
- IT (Italy)
- KR (Korea)
- CN (China)
- Nation
- World
- Business
- Politics
- Science
- Technology
- Entertainment
- Sports
It is useful to have a pull-down menu to select the country and category.
Setting up pull-down menus prevents input errors and makes it easy to make changes.
How to create a pull-down menu
- Select the cell for which you want to create a Dropdown menu (e.g., cell B1).
- Right-click and select “Dropdown” from the menu that appears
- Enter the country code in the “Data validation rules” item field displayed on the right side of the screen.
- Select “Add another item” to add other country codes as well.
- When you are finished, click Done.
Google Apps Script Execution
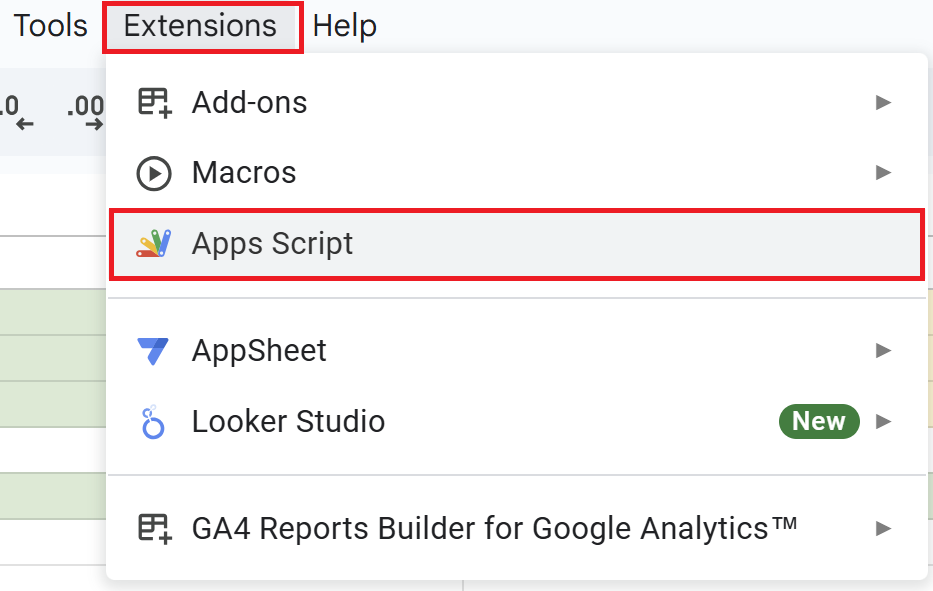
Open a Google Spreadsheet and click on “Extensions” in the menu, then “Apps Script” to open the Apps Script editor.
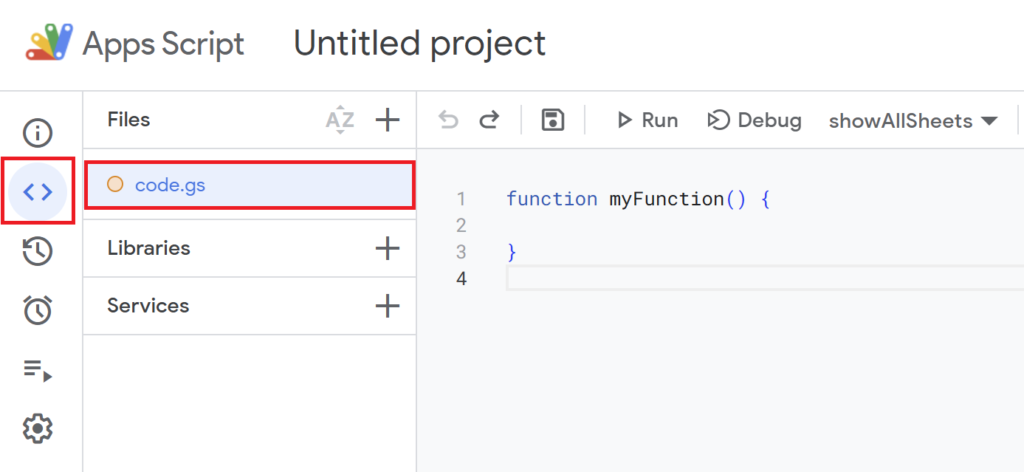
function myFunction(){
}
is included from the beginning, so delete it and paste the previous script.
function fetchGoogleNewsByCountryAndSection() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("News");
// Retrieve country, section, and keyword values from the spreadsheet
const country = sheet.getRange("B1").getValue().toString().toUpperCase(); // Example: "JP"
const section = sheet.getRange("B2").getValue().toString().toUpperCase(); // Example: "WORLD"
const keyword = sheet.getRange("B3").getValue().toString(); // Example: "Technology"
// Set the language based on the country code
let language = 'en'; // Default is English
if (country === 'JP') {
language = 'ja';
} else if (country === 'FR') {
language = 'fr';
} else if (country === 'DE') {
language = 'de';
} else if (country === 'ES') {
language = 'es';
} else if (country === 'IT') {
language = 'it';
} else if (country === 'KR') {
language = 'ko';
} else if (country === 'CN') {
language = 'zh-CN';
}
// Construct the RSS feed URL
const rssUrl = `https://news.google.com/rss/headlines/section/topic/${section}?hl=${language}&gl=${country}&ceid=${country}:${language}`;
// Fetch the RSS feed
const response = UrlFetchApp.fetch(rssUrl);
const xml = XmlService.parse(response.getContentText());
const root = xml.getRootElement();
const channel = root.getChild("channel");
const items = channel.getChildren("item");
// Clear existing news data starting from row 6 (columns A to C)
if (sheet.getLastRow() > 5) {
sheet.getRange(6, 1, sheet.getLastRow() - 5, 3).clearContent(); // Clear columns A to C
}
// Write news article data (title, link, publication date) to the sheet
let rowIndex = 6;
items.forEach(item => {
const title = item.getChildText("title");
const link = item.getChildText("link");
const pubDate = item.getChildText("pubDate");
// Keyword filtering (only apply if a keyword is provided)
if (keyword && !title.includes(keyword)) {
return; // Skip if the title does not contain the keyword
}
sheet.getRange(rowIndex, 1).setValue(title); // Title (column A)
sheet.getRange(rowIndex, 2).setValue(link); // Link (column B)
sheet.getRange(rowIndex, 3).setValue(pubDate); // Publication date (column C)
rowIndex++;
});
}
Script Description
- Retrieve Country, Category and Keywords
-
function fetchGoogleNewsByCountryAndSection() { const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("News"); // Retrieve country, section, and keyword values from the spreadsheet const country = sheet.getRange("B1").getValue().toString().toUpperCase(); // Example: "JP" const section = sheet.getRange("B2").getValue().toString().toUpperCase(); // Example: "WORLD" const keyword = sheet.getRange("B3").getValue().toString(); // Example: "Technology"
- Refer to the spreadsheet sheet name “News”.
- Get the country (e.g., JP), category (e.g., WORLD), and keyword (e.g., technology) from the B1, B2, and B3 cells in the spreadsheet.
- Obtains the country code and determines the language to be displayed based on it (e.g., English for US, Japanese for JP).
- Composition of RSS Feed
-
// Set the language based on the country code let language = 'en'; // Default is English if (country === 'JP') { language = 'ja'; } else if (country === 'FR') { language = 'fr'; } else if (country === 'DE') { language = 'de'; } else if (country === 'ES') { language = 'es'; } else if (country === 'IT') { language = 'it'; } else if (country === 'KR') { language = 'ko'; } else if (country === 'CN') { language = 'zh-CN'; } // Construct the RSS feed URL const rssUrl = `https://news.google.com/rss/headlines/section/topic/${section}?hl=${language}&gl=${country}&ceid=${country}:${language}`;
- Create a Google News RSS feed URL based on the country and category information retrieved.
- By dynamically setting the hl (language) and gl (region) parameters, news from a specific country can be retrieved in a specific language.
- Retrieving News Articles
-
// Fetch the RSS feed const response = UrlFetchApp.fetch(rssUrl); const xml = XmlService.parse(response.getContentText()); const root = xml.getRootElement(); const channel = root.getChild("channel"); const items = channel.getChildren("item");
- Use the
UrlFetchApp.fetch()
function to retrieve news data from the RSS feed URL you created. - The data retrieved in XML format is parsed to extract news titles, links, publication dates, etc.
- Use the
- Writing to Spreadsheets
-
// Write news article data (title, link, publication date) to the sheet let rowIndex = 6; items.forEach(item => { const title = item.getChildText("title"); const link = item.getChildText("link"); const pubDate = item.getChildText("pubDate"); // Keyword filtering (only apply if a keyword is provided) if (keyword && !title.includes(keyword)) { return; // Skip if the title does not contain the keyword } sheet.getRange(rowIndex, 1).setValue(title); // Title (column A) sheet.getRange(rowIndex, 2).setValue(link); // Link (column B) sheet.getRange(rowIndex, 3).setValue(pubDate); // Publication date (column C) rowIndex++; }); }
- Write the retrieved news article data in cell A6 and after in the spreadsheet.
- Existing data is cleared and newly acquired news information is written in sequence.
- If a keyword is set, only articles that contain that keyword in the news title will be displayed.
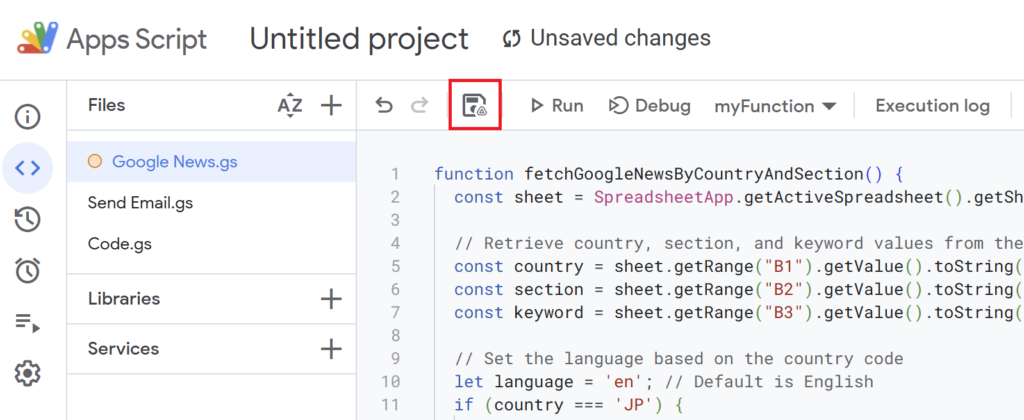
After writing the script, name and save it.
(e.g., “Google News”)
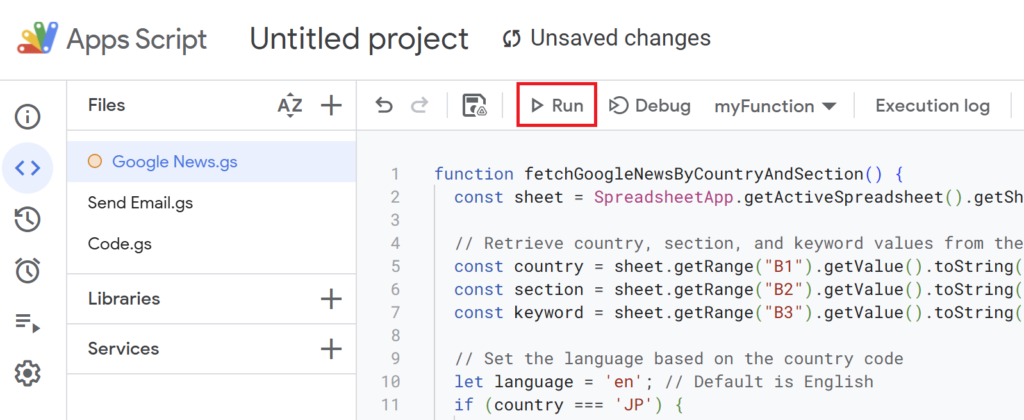
Run the script to get the news.
If you’re running the script for the first time, you need to authorize it.
Therefore, press “Review Permissions.“
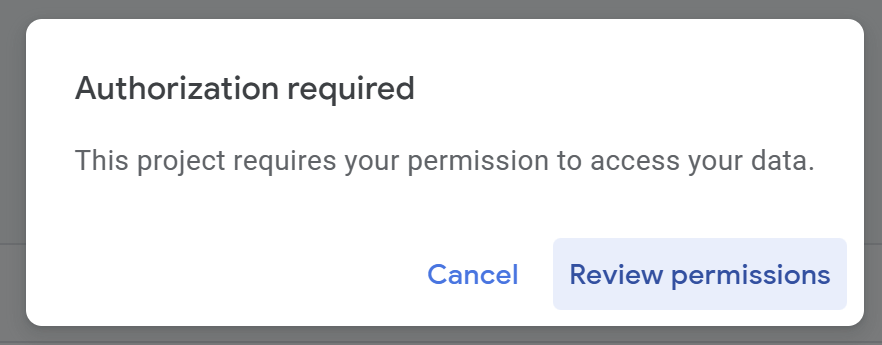
Detailed Authorization Steps
Press “Advanced.”
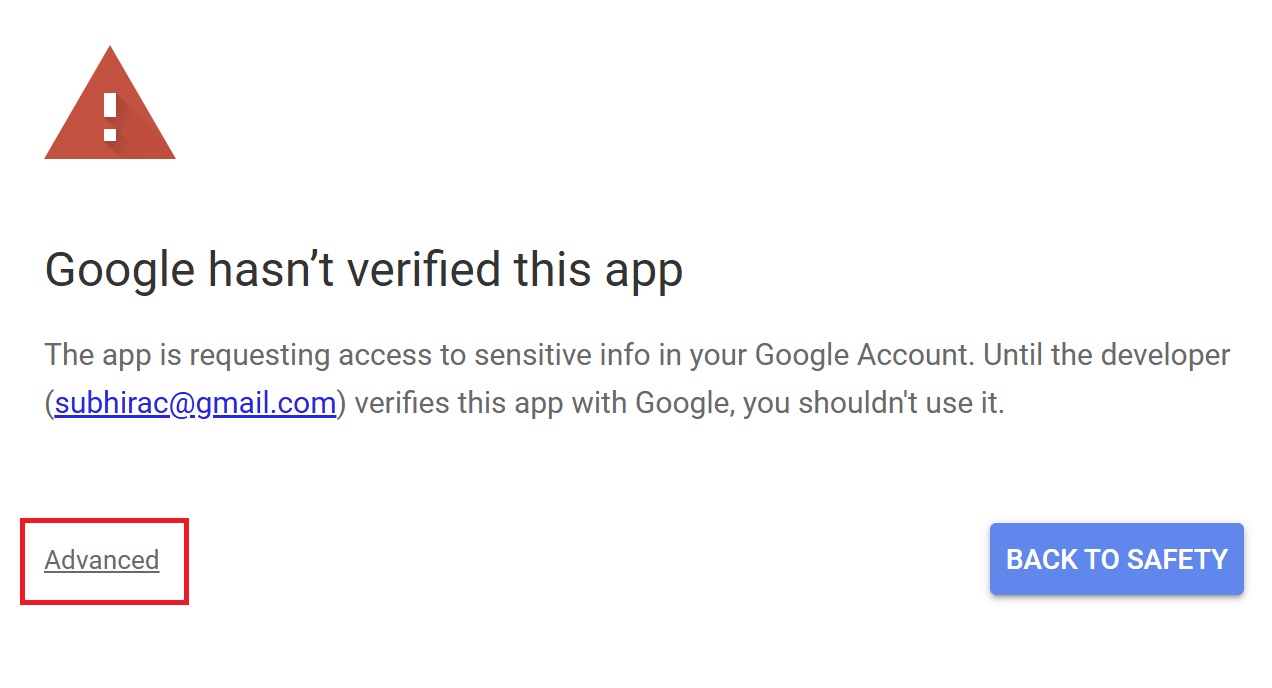
Press “Go to Untitled project (Unsafe).”
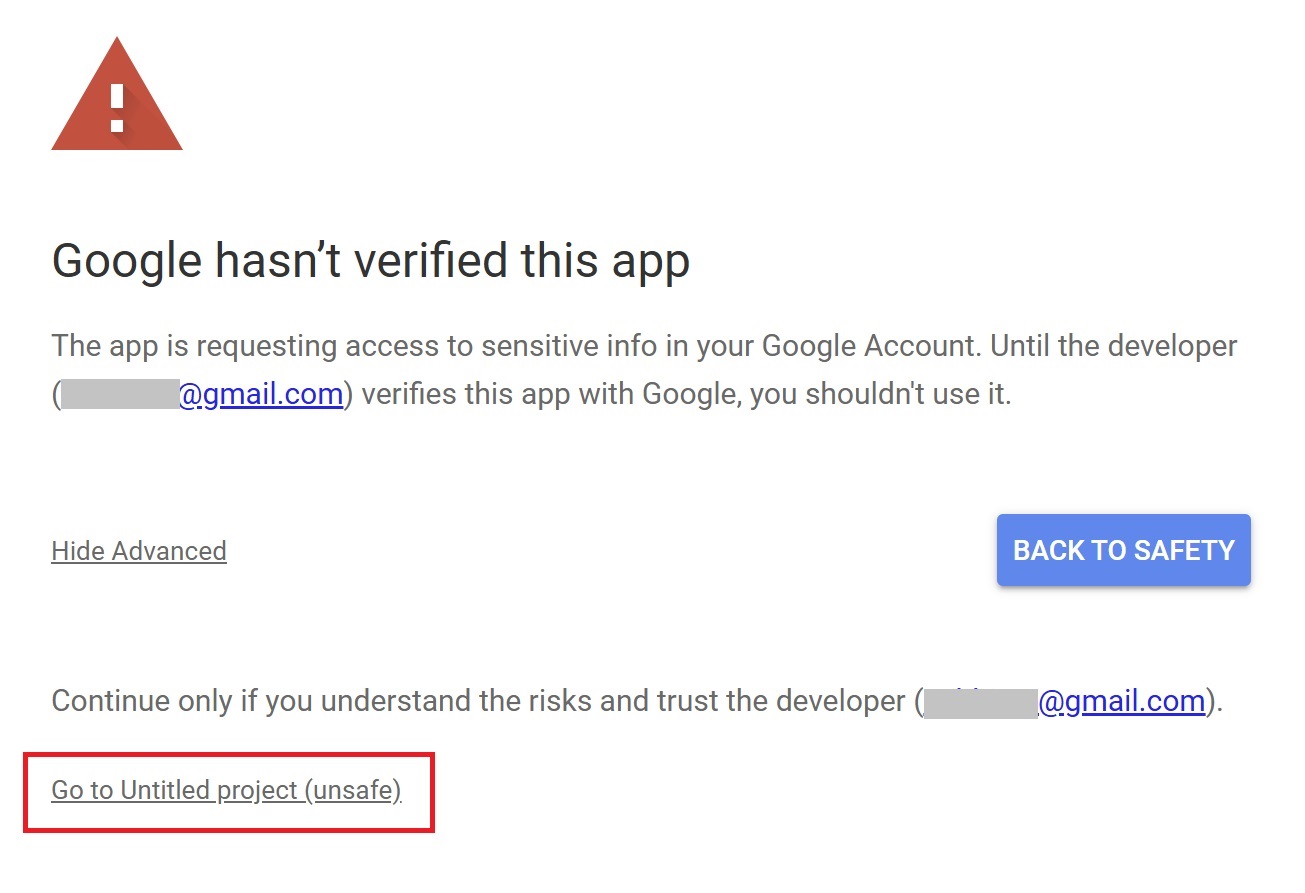
After that, press “Allow.”
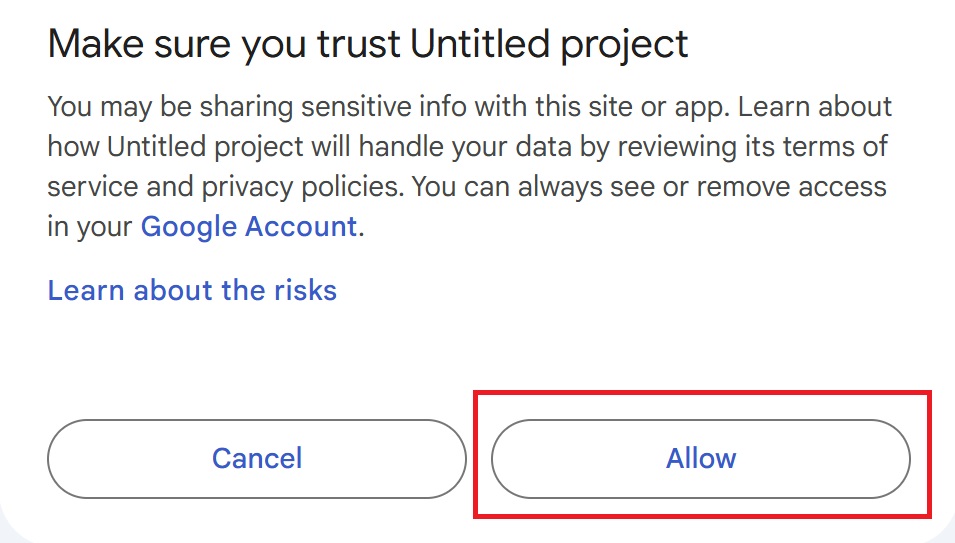
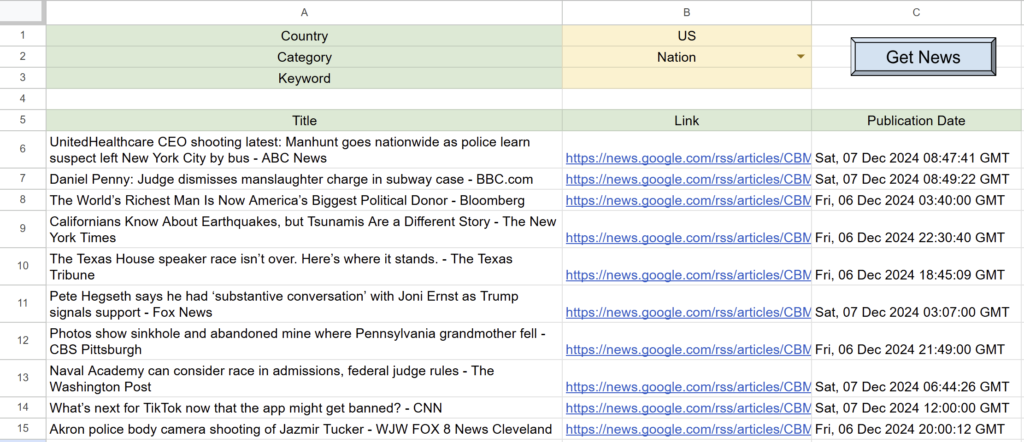
The news will be reflected in the spreadsheet after the fifth line.
If you want to change the country or category and run the script repeatedly, place a button for the script to make it easier to retrieve.
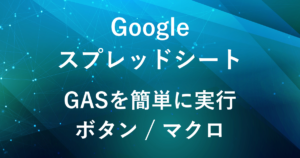
Automatic collection at a fixed timing by setting triggers
To retrieve news automatically, set a “trigger”.
This will automatically run the script at the specified time to retrieve the latest news information.
How to Set Trigger
The same procedure as before.
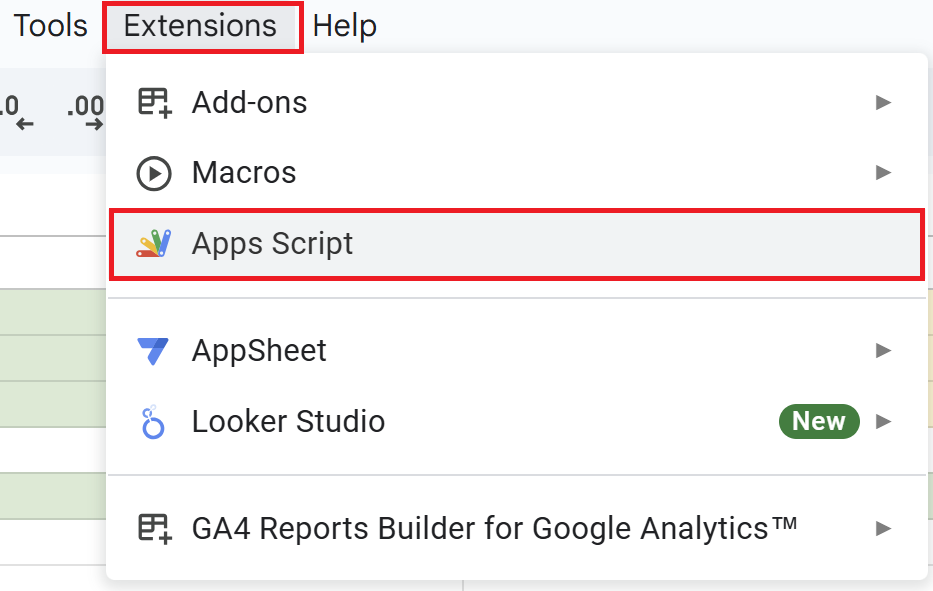
Open a Google Spreadsheet and click on “Extensions” in the menu, then “Apps Script” to open the Apps Script editor.
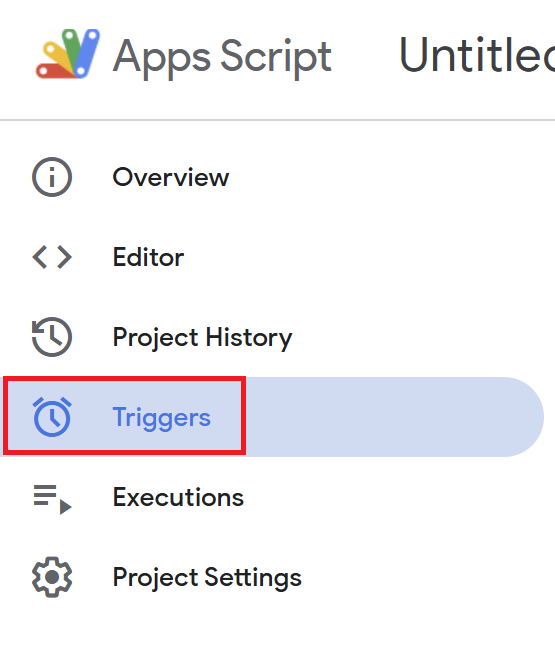
Click on the trigger icon (clock icon).
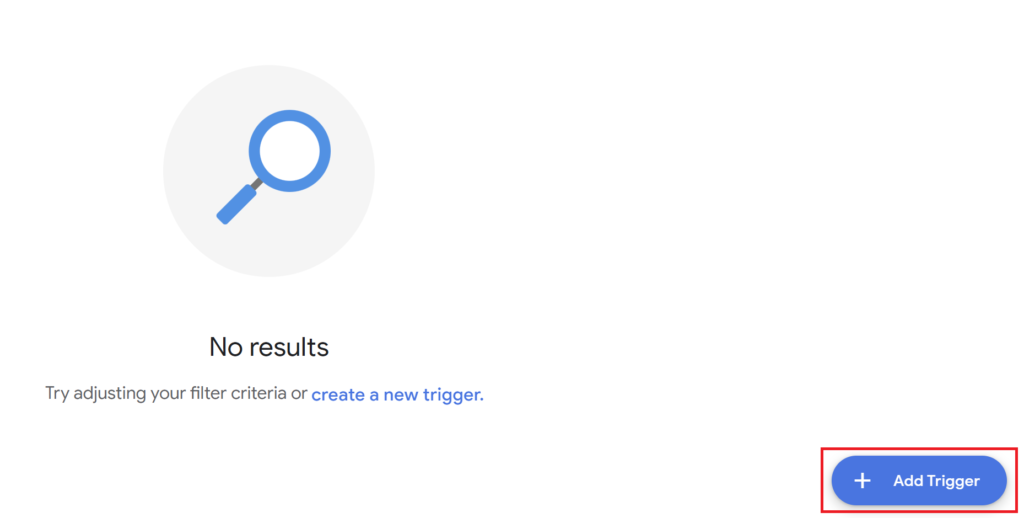
Press the “Add Trigger” button and configure as follows
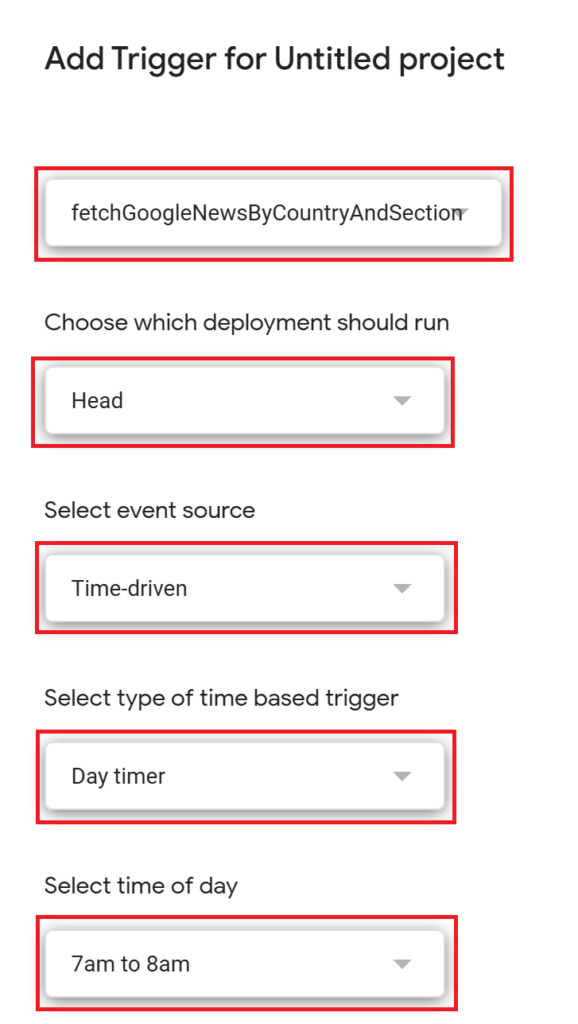
- Select a function :
fetchGoogleNewsByCountryAndSection
- Select execution deployment:
Head
- Select event source: 「Time-driven」
- Time-based trigger: 「Day timer」
- Select time: For example, you can set “7am to 8am” to get news automatically every morning.
Conclusion
This Google Apps Script eliminates the need to manually visit news sites.
In addition, articles can be filtered by specific keywords to efficiently retrieve news information.
We hope you will take advantage of it.
-
How to Bulk Create Shortened URLs Using Google Sheets and Google Apps Script
-
Bulk Delete Files in Google Drive with Google Apps Script: Organize with Spreadsheets
-
How to Efficiently Organize Business Card Data with ChatGPT API: Leveraging Google Sheets and AI
Our company offers support for improving work efficiency through the use of Google Apps Script.
If you need assistance with Google Apps Script customization or error resolution, please feel free to contact us.
We are fully committed to supporting your business improvements.
Contact us here
Comments