How to Efficiently Organize Business Card Data with ChatGPT API: Leveraging Google Sheets and AI
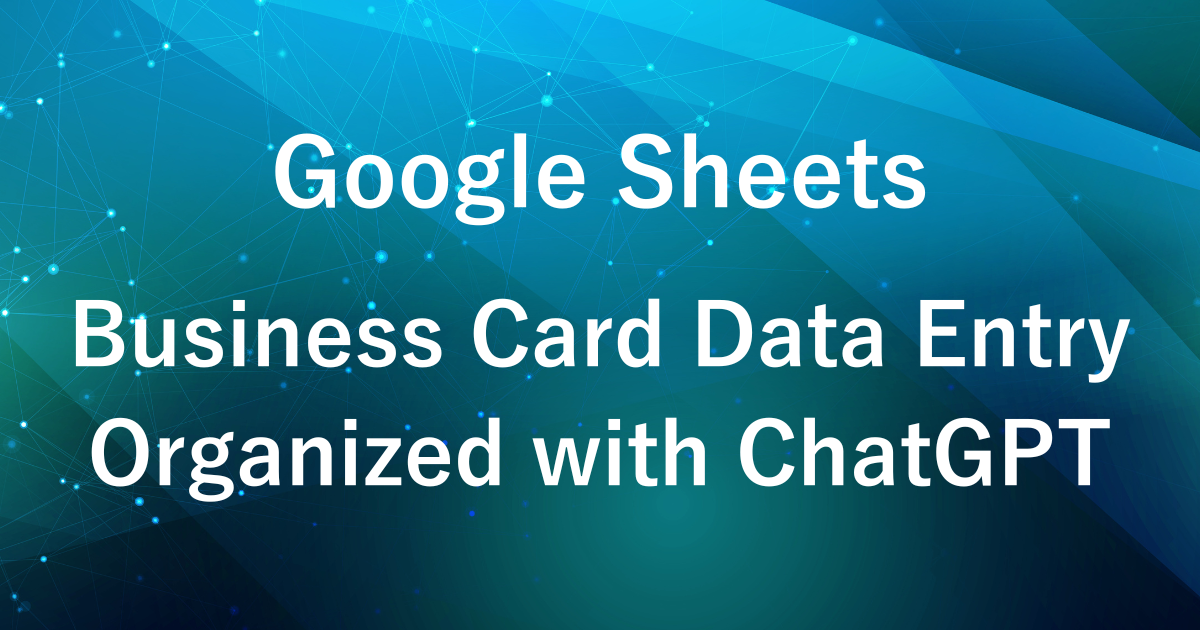
- Those who spend too much time manually inputting business card information.
- Those who want to automate business card data entry and streamline their workflow.
In the previous article, we demonstrated how to use OCR (Optical Character Recognition) and GAS to transfer business card data into a Google Spreadsheet.
However, in that setup, the entire business card information is stored in Column A.
In this article, we’ll show you how to use the ChatGPT API to organize the data in Column A into specific fields, such as Company Name, Name, Job Title, Phone Number, and more.
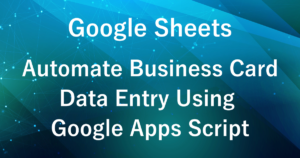
Example Output
Before Data Organization
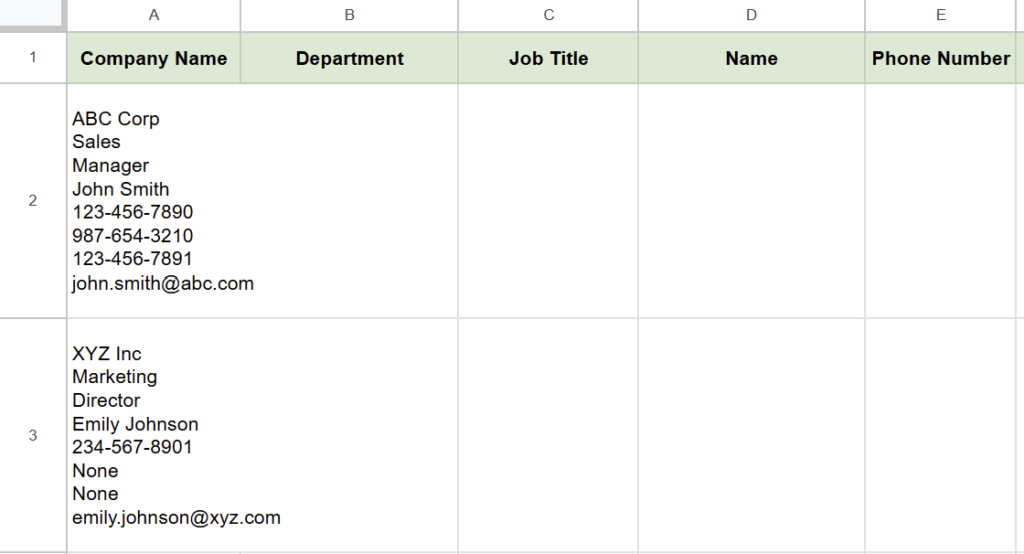
After Data Organization (Using ChatGPT API)

After the business card information is transferred to Column A, the ChatGPT API is used to organize the data into specific fields.
The spreadsheet includes columns such as “Company Name,” “Department,” “Name,” and “Job Title,” where the corresponding data is automatically input into the appropriate columns.
Preparing the ChatGPT API
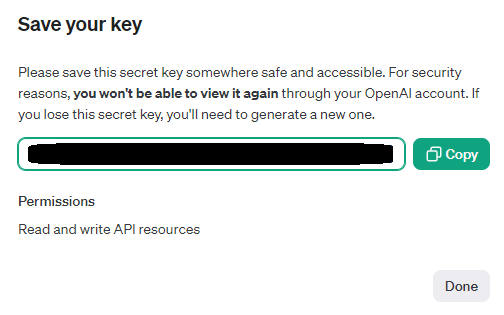
Before creating the GAS script, you need to obtain a ChatGPT API key.
For detailed instructions on how to obtain the API key, please refer to a separate article.
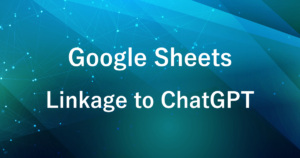
Steps
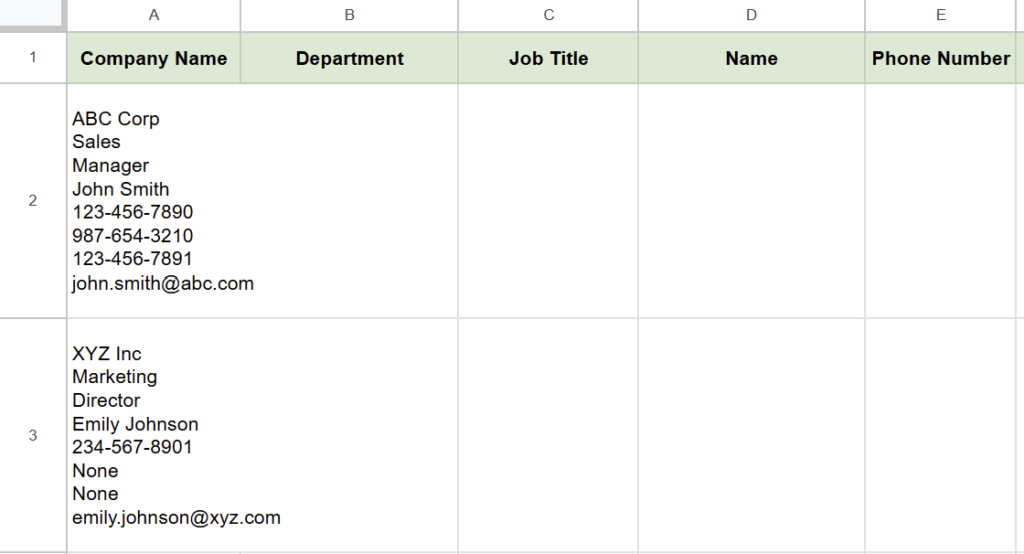
We will use a spreadsheet containing business card information that has already been transferred.
For details on how to extract and transfer business card information, please refer to the following article.
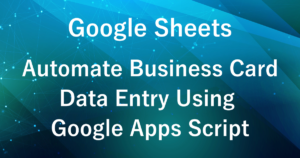
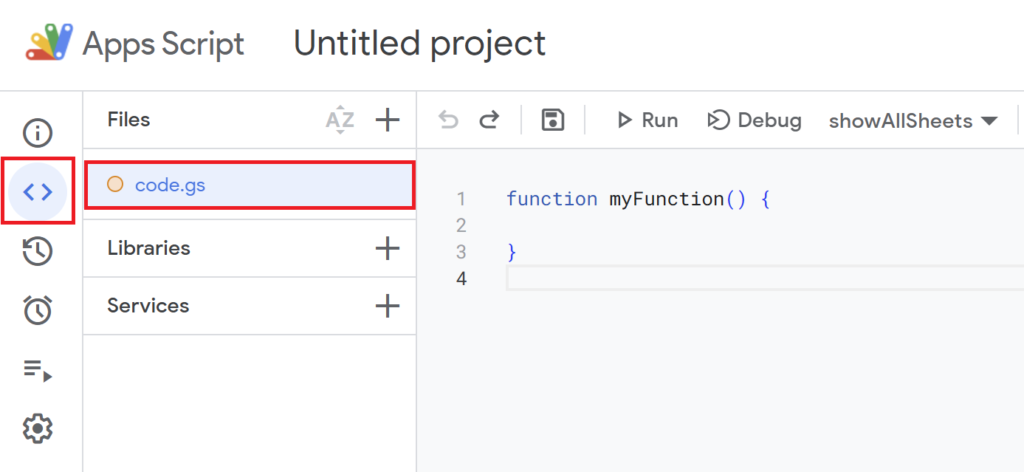
Since the default function myFunction() {}
is present, delete it and replace it with the script below.
// Google Apps Script to Use ChatGPT API for Parsing Business Card Information
// Function to parse business card information
function parseBusinessCard() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
const dataRange = sheet.getRange("A2:A" + sheet.getLastRow());
const data = dataRange.getValues();
data.forEach((row, index) => {
const cardInfo = row[0];
const rowIndex = index + 2; // Adjust for the header row
const isRowAlreadyParsed = sheet.getRange(`B${rowIndex}:L${rowIndex}`).getValues()[0].some(cell => cell !== "");
// Process only rows with data in Column A and not already parsed
if (cardInfo && !isRowAlreadyParsed) {
const parsedData = getChatGPTParsedData(sheet, cardInfo);
updateSheetWithParsedData(sheet, rowIndex, parsedData);
}
});
}
// Function to retrieve parsed data from ChatGPT API
function getChatGPTParsedData(sheet, cardInfo) {
const apiKey = PropertiesService.getScriptProperties().getProperty("OPENAI_API_KEY");
const url = "https://api.openai.com/v1/chat/completions";
const headers = sheet.getRange("A1:L1").getValues()[0];
const headersJson = headers.reduce((acc, header) => {
acc[header] = ""; // Initialize JSON structure with empty values
return acc;
}, {});
const messages = [
{
"role": "user",
"content": `Please parse the following business card information into the specified JSON format: ${JSON.stringify(headersJson)}: ${cardInfo}`
}
];
const options = {
"method": "post",
"headers": {
"Authorization": `Bearer ${apiKey}`,
"Content-Type": "application/json"
},
"payload": JSON.stringify({
"model": "gpt-4",
"messages": messages,
"max_tokens": 500,
"temperature": 0.7
})
};
try {
const response = UrlFetchApp.fetch(url, options);
const json = JSON.parse(response.getContentText());
const parsedData = JSON.parse(json.choices[0].message.content.trim());
return parsedData;
} catch (error) {
Logger.log("Error while fetching data from ChatGPT API: " + error);
return null;
}
}
// Function to update the spreadsheet with parsed data
function updateSheetWithParsedData(sheet, rowIndex, parsedData) {
if (parsedData) {
const headers = sheet.getRange("A1:L1").getValues()[0];
headers.forEach((header, index) => {
sheet.getRange(rowIndex, index + 1).setValue(parsedData[header] || ""); // Populate columns with parsed data
});
}
}
// Function to set the ChatGPT API key in script properties
function setApiKey() {
const apiKey = "YOUR_API_KEY_HERE";
PropertiesService.getScriptProperties().setProperty("OPENAI_API_KEY", apiKey);
}
Script Description
- Script Overview
-
This script uses the ChatGPT API to analyze business card information summarized in a Google Spreadsheet and classify it into specific fields, such as Company Name, Department, Job Title, and Name. The classified data is then entered into their respective columns in the spreadsheet.
The business card information is initially stored in a single cell, separated by line breaks. When the script is executed, each piece of information is distributed to the corresponding fields.
Additionally, rows that have already been classified are skipped to avoid duplicate processing.
For this example, the ChatGPT model and token count are configured as follows:
- ChatGPT Model Used: GPT-4
- Maximum Token Count: 500
parseBusinessCard()
Function: Analyze Business Card Information-
// Function to parse business card information function parseBusinessCard() { const sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet(); const dataRange = sheet.getRange("A2:A" + sheet.getLastRow()); const data = dataRange.getValues(); data.forEach((row, index) => { const cardInfo = row[0]; const rowIndex = index + 2; // Adjust for the header row const isRowAlreadyParsed = sheet.getRange(`B${rowIndex}:L${rowIndex}`).getValues()[0].some(cell => cell !== ""); // Process only rows with data in Column A and not already parsed if (cardInfo && !isRowAlreadyParsed) { const parsedData = getChatGPTParsedData(sheet, cardInfo); updateSheetWithParsedData(sheet, rowIndex, parsedData); } }); }
dataRange
: Retrieves data from Column A in the spreadsheet (from A2 to the last row).data.forEach
: Iterates through each row of data in Column A and processes it sequentially.isRowAlreadyParsed
: Skips rows if any cells in Columns B to L already contain data.getChatGPTParsedData()
: Calls the ChatGPT API and returns the business card information as a JSON object, with the data categorized into specific fields.updateSheetWithParsedData()
: Updates the spreadsheet with the parsed data from the API response.
getChatGPTParsedData(sheet, cardInfo)
Function: Retrieve Parsed Data from the ChatGPT API-
// Function to retrieve parsed data from ChatGPT API function getChatGPTParsedData(sheet, cardInfo) { const apiKey = PropertiesService.getScriptProperties().getProperty("OPENAI_API_KEY"); const url = "https://api.openai.com/v1/chat/completions"; const headers = sheet.getRange("A1:L1").getValues()[0]; const headersJson = headers.reduce((acc, header) => { acc[header] = ""; // Initialize JSON structure with empty values return acc; }, {}); const messages = [ { "role": "user", "content": `Please parse the following business card information into the specified JSON format: ${JSON.stringify(headersJson)}: ${cardInfo}` } ]; const options = { "method": "post", "headers": { "Authorization": `Bearer ${apiKey}`, "Content-Type": "application/json" }, "payload": JSON.stringify({ "model": "gpt-4", "messages": messages, "max_tokens": 500, "temperature": 0.7 }) }; try { const response = UrlFetchApp.fetch(url, options); const json = JSON.parse(response.getContentText()); const parsedData = JSON.parse(json.choices[0].message.content.trim()); return parsedData; } catch (error) { Logger.log("Error while fetching data from ChatGPT API: " + error); return null; } }
headers
: Retrieves the headers (field names) from the first row (A1) of the spreadsheet. These headers define the format for categorizing the data.messages
: Creates the message to be sent to the API. This message includes instructions to categorize the business card information into JSON format based on the specified fields.UrlFetchApp.fetch()
: Sends a request to the ChatGPT API and retrieves the analysis results.parsedData
: Parses the API response into JSON format and returns the business card information categorized into specific fields.
updateSheetWithParsedData(sheet, rowIndex, parsedData)
Function: Update the Spreadsheet with Parsed Data-
// Function to update the spreadsheet with parsed data function updateSheetWithParsedData(sheet, rowIndex, parsedData) { if (parsedData) { const headers = sheet.getRange("A1:L1").getValues()[0]; headers.forEach((header, index) => { sheet.getRange(rowIndex, index + 1).setValue(parsedData[header] || ""); // Populate columns with parsed data }); } }
headers
: Retrieves the header fields from the first row of the spreadsheet. These headers are used to determine where to input the parsed data in the corresponding columns.sheet.getRange().setValue()
: Inputs the parsed data into the appropriate cells in the spreadsheet based on the headers.
setApiKey()
Function to Set the ChatGPT API Key in Script Properties-
// Function to set the ChatGPT API key in script properties function setApiKey() { const apiKey = "YOUR_API_KEY_HERE"; PropertiesService.getScriptProperties().setProperty("OPENAI_API_KEY", apiKey); }
- This function is used to save the ChatGPT API key into the script properties.
- By setting the API key, you enable the script to communicate with the ChatGPT API.
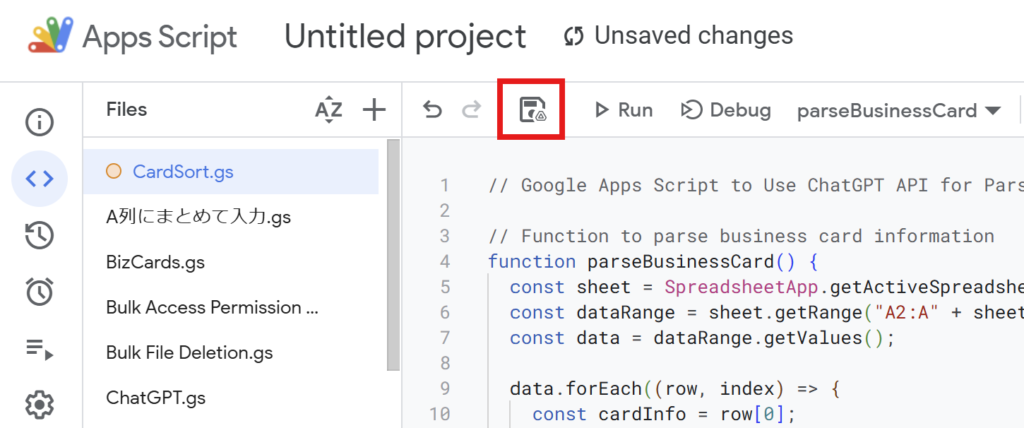
Once you’ve written the code, save it with an appropriate name.
Example: CardSort
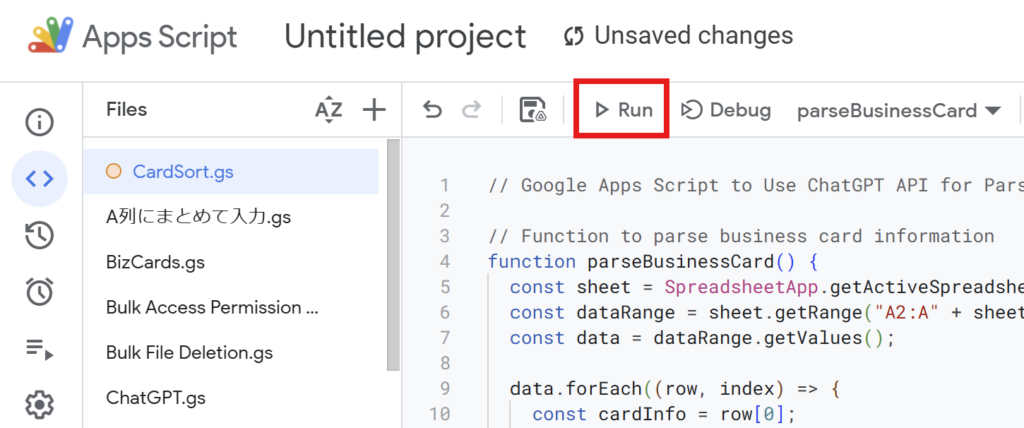
Run the script to distribute the business card data from Column A into the corresponding columns for each field.
If you’re running the script for the first time, you need to authorize it.
Therefore, press “Review Permissions.“
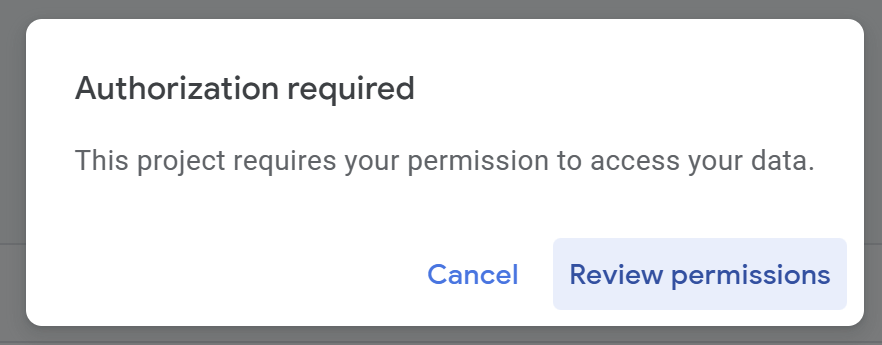
Detailed Authorization Steps
Press “Advanced.”
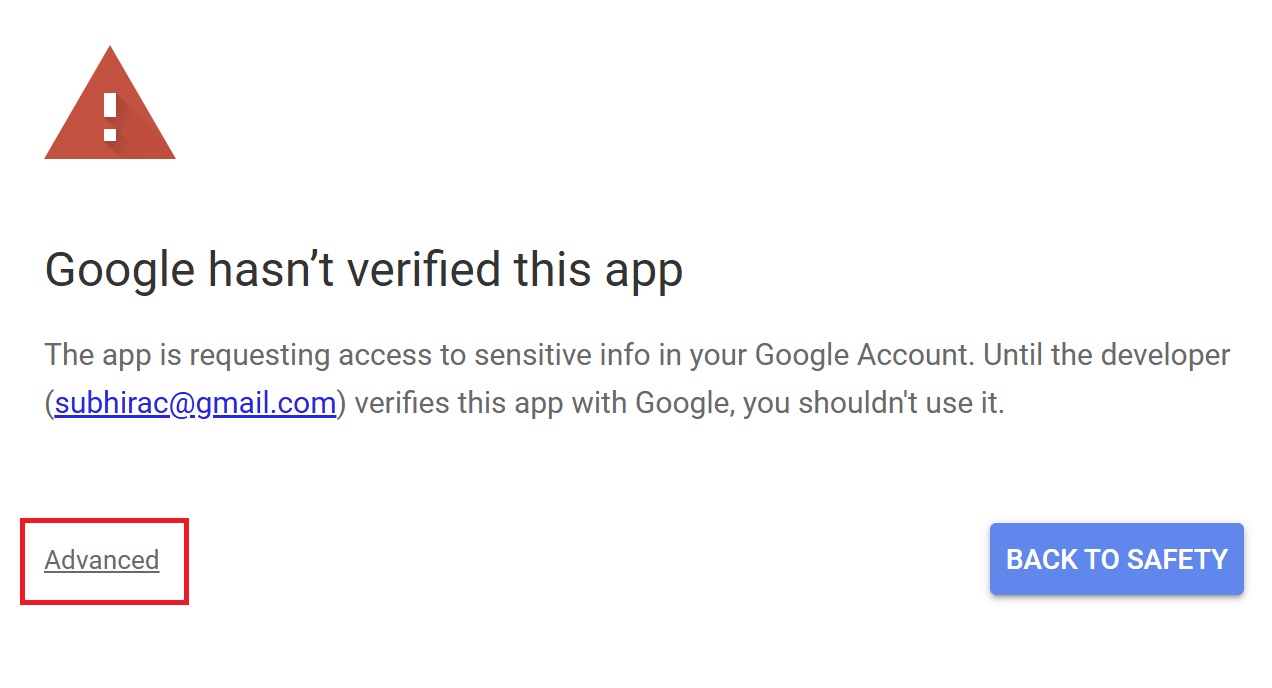
Press “Go to Untitled project (Unsafe).”
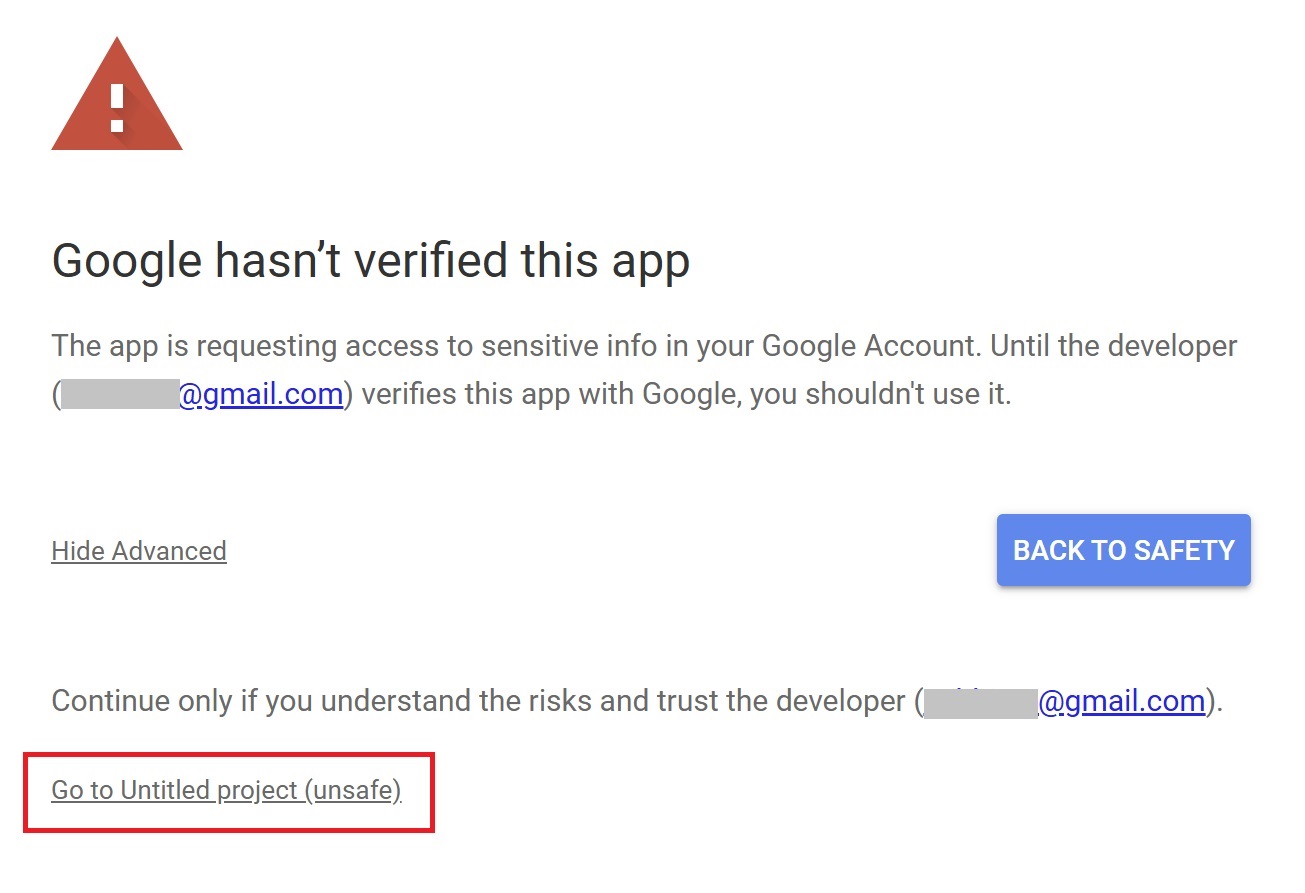
After that, press “Allow.”
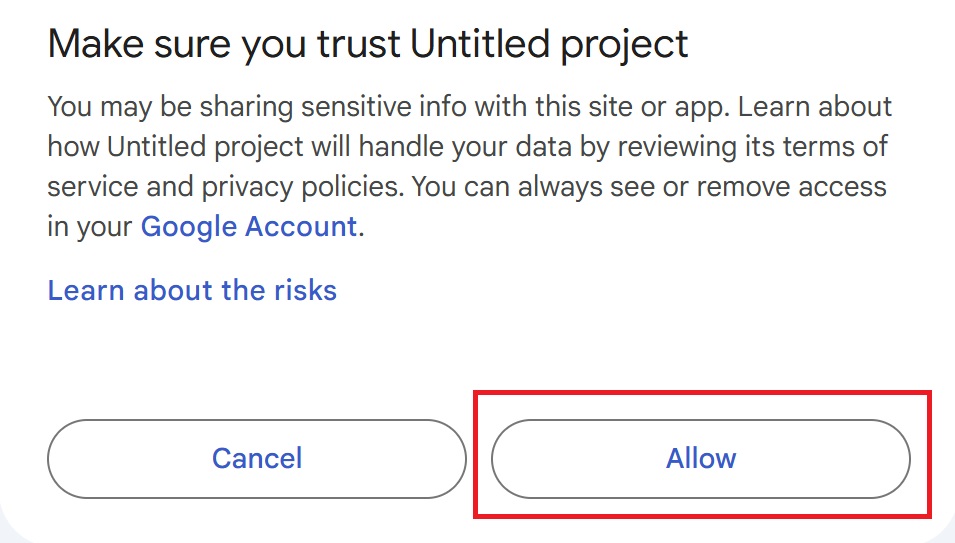
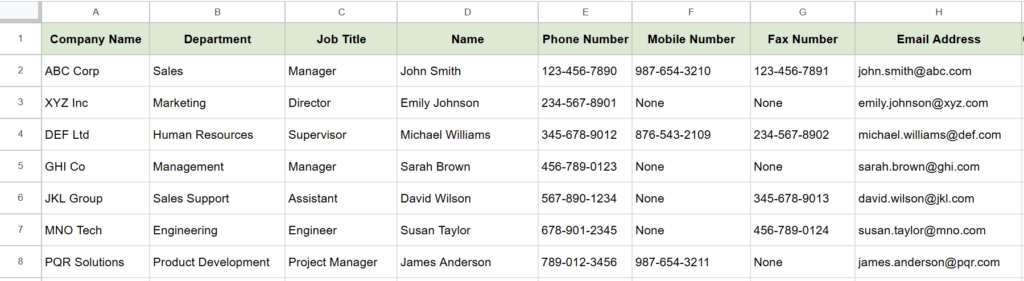
The business card data will be categorized and input into the corresponding fields.
Afterward, review the entered data manually to ensure there are no issues.
Conclusion
In this article, we demonstrated how to use the ChatGPT API to distribute business card data from Column A of a spreadsheet into specific fields.
While the final categorized data should be reviewed manually for accuracy, this method allows you to efficiently organize business card information.
The approach using the ChatGPT API can be applied not only to business card management but also to other categorization tasks, making it a versatile tool for data organization.
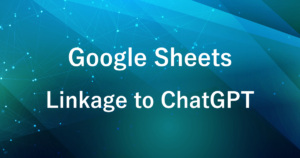
-
Master XLOOKUP: Simplify Data Search and Learn the Key Differences from VLOOKUP and HLOOKUP
-
Enhance User Interaction with Google Apps Script: How to Add Confirmation Pop-Ups to Prevent Mistakes
-
Automate News Collection with Google Apps Script: Fetch Daily Updates from Google News to Google Sheets
Our company offers support for improving work efficiency through the use of Google Apps Script.
If you need assistance with Google Apps Script customization or error resolution, please feel free to contact us.
We are fully committed to supporting your business improvements.
Contact us here
Comments