How to Bulk Create Shortened URLs Using Google Sheets and Google Apps Script
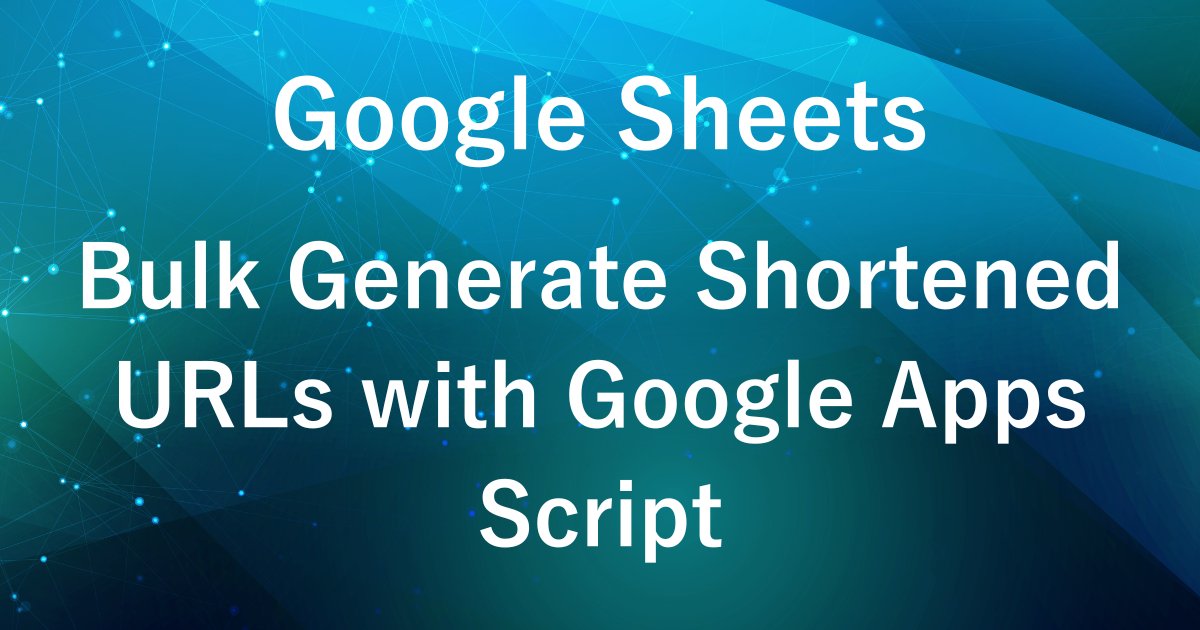
In our previous article (“Simplify URL Shortening: Create and Copy Short URLs with a Single Click Using Chrome Extensions“), we introduced how to create shortened URLs using a Chrome extension, which is perfect for converting URLs individually.
However, if you need to shorten a large number of URLs, using Google Apps Script (GAS) is more efficient.
In this article, we’ll show you how to use Google Sheets and Google Apps Script to bulk convert multiple URLs into shortened URLs.
By creating and managing shortened URLs collectively in a Google Spreadsheet, you can streamline your workflow and enhance productivity.
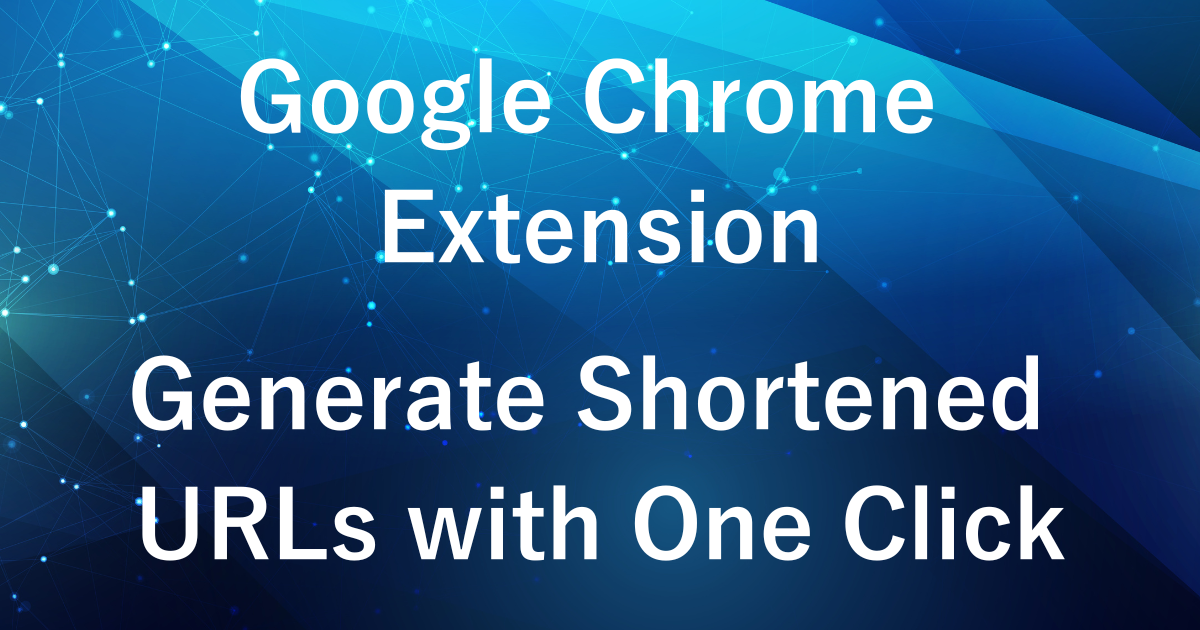
Example Output
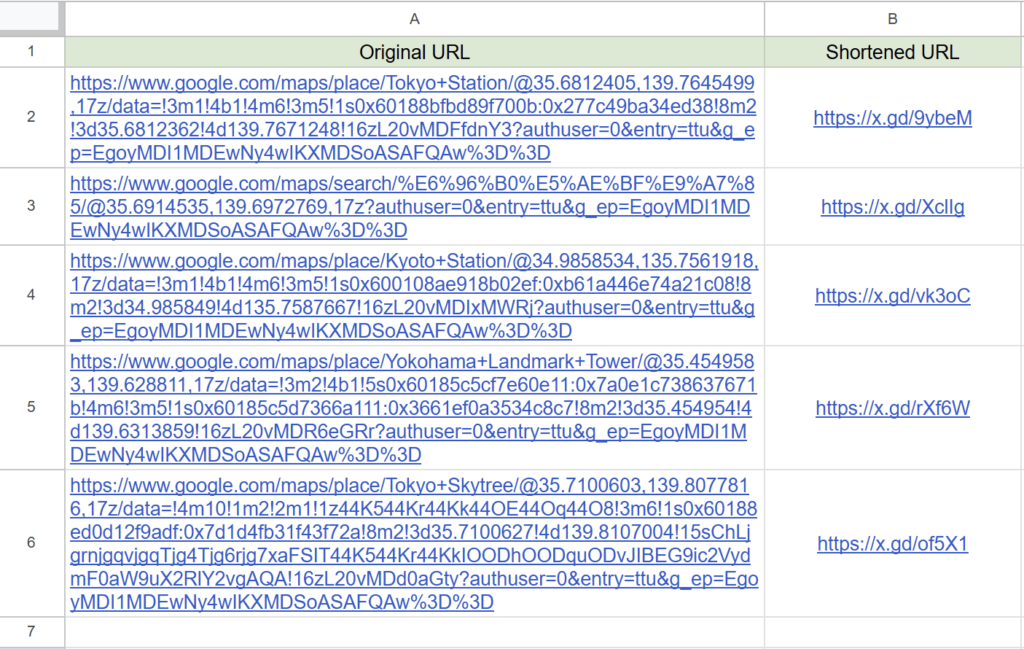
- Using Google Apps Script and the X.gd API
- Enter the original URLs to be shortened in column A
- The shortened URLs generated by Google Apps Script will appear in column B
Bulk Create Shortened URLs from Original URLs Entered in Column A
The script is designed to skip URLs that already have shortened versions in column B, processing only the URLs that have not yet been shortened.
This approach ensures efficient URL creation by eliminating unnecessary processing.
Preparation Needed
To bulk shorten multiple URLs, you need to obtain an API key from X.gd.
Follow the steps below to acquire your API key and set it up for use in Google Sheets.
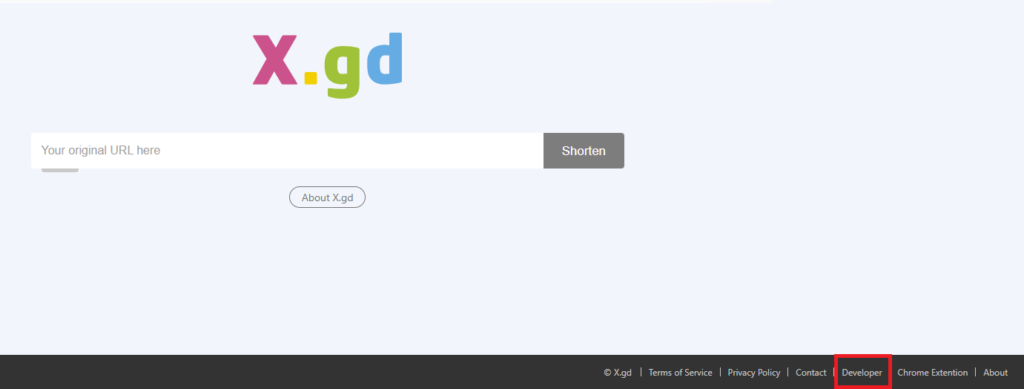
Click on the “Developer” page located at the bottom right of the website.
Here, you will find the procedure to issue an API key.
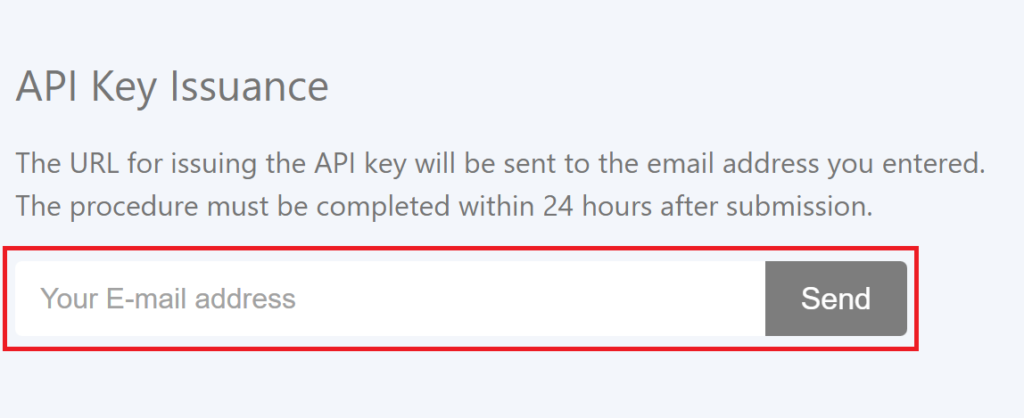
Scroll down the Developers page to find the section for issuing an API key.
Follow the instructions to submit your email address.
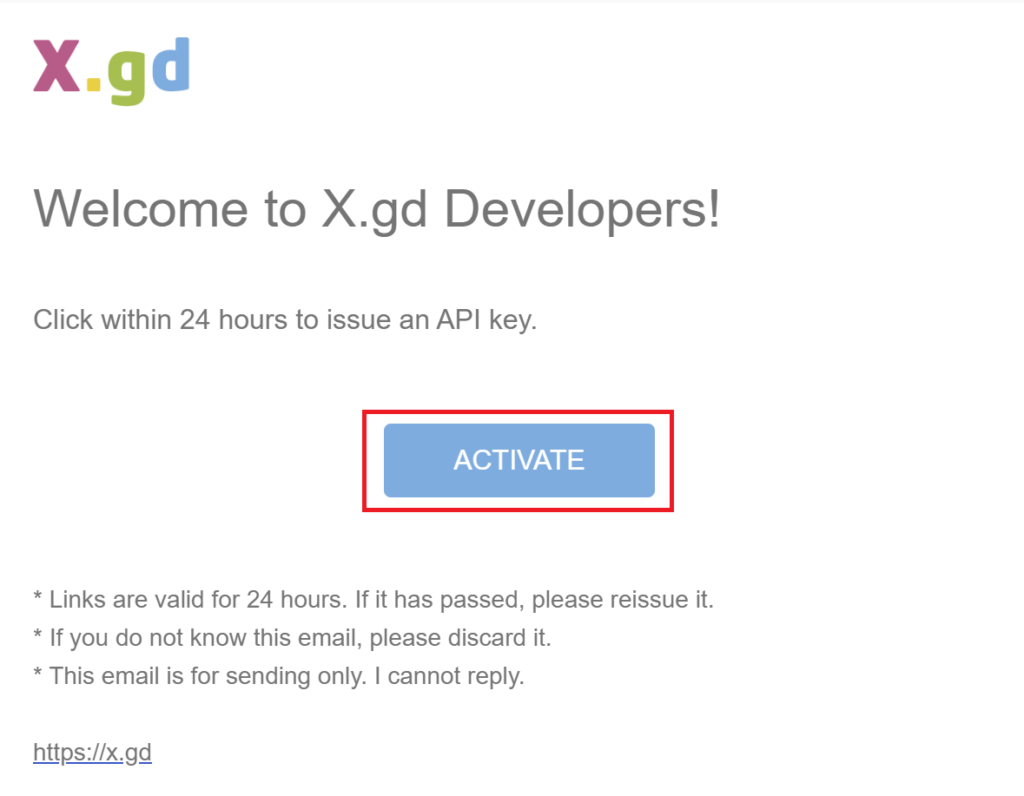
You will receive a confirmation email from X.gd at the email address you provided.
Click the “Activate” button in the email to activate your API key.
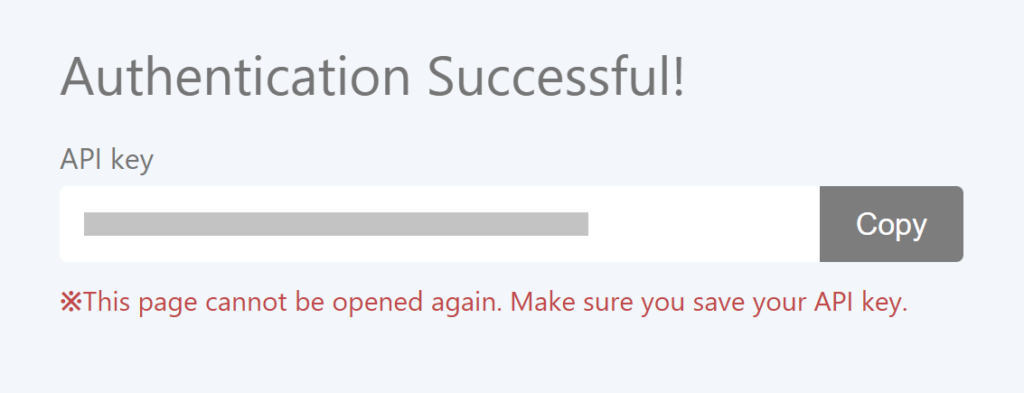
After clicking “Activate”, your API key will be displayed.
Copy this key, as you will need to set it in the script properties in a later step.
Steps
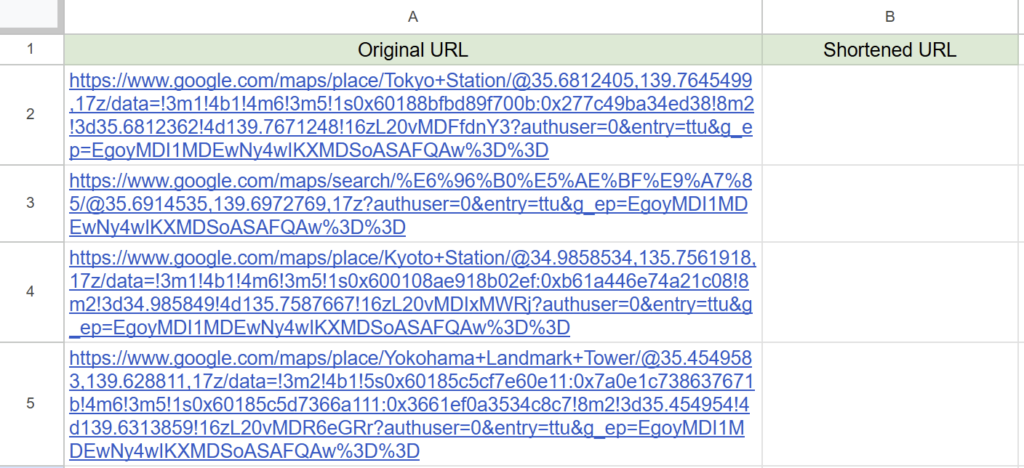
- Enter the Headers
-
- Column A: Original URL
- Column B: Shortened URL
- Input the URLs to be Shortened
-
Enter the URLs you want to shorten in column A, starting from row 2.
- Set the Sheet Name
-
Rename the sheet to “Shortened URLs”, as it will be referenced during the execution of the Apps Script.
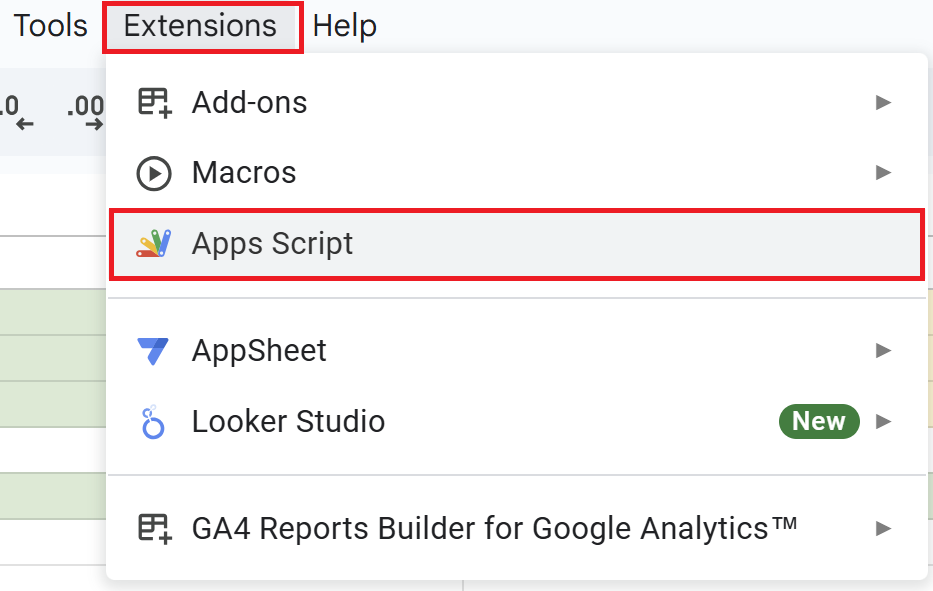
Open a Google Spreadsheet and click on “Extensions” in the menu, then “Apps Script” to open the Apps Script editor.
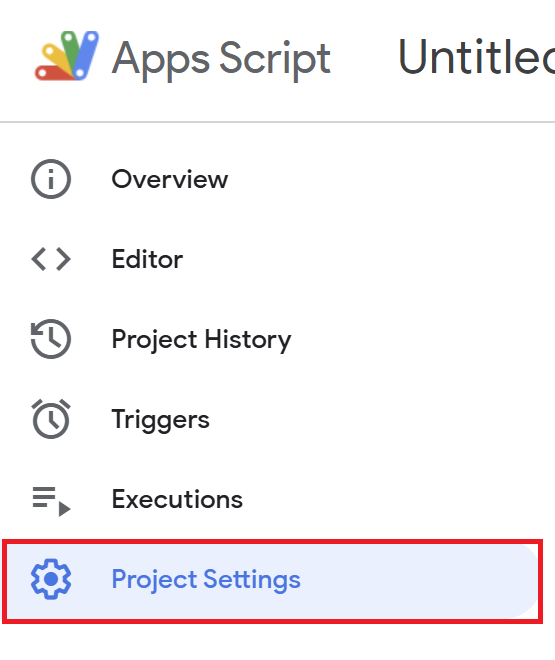
Open the Google Apps Script Editor and select “Project Settings”.
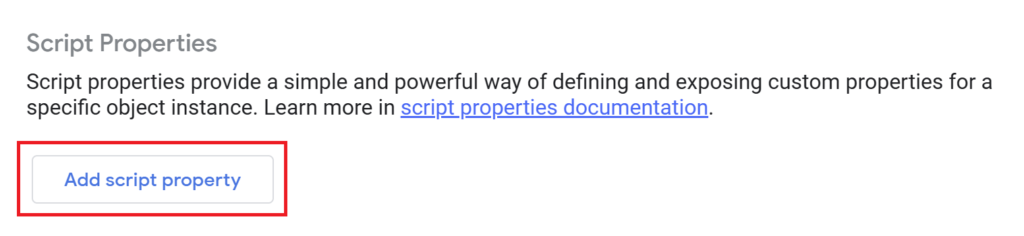
Choose “Add Script Property”, and enter a key name (e.g., "URL_SHORTENER_API_KEY"
) and your API key.
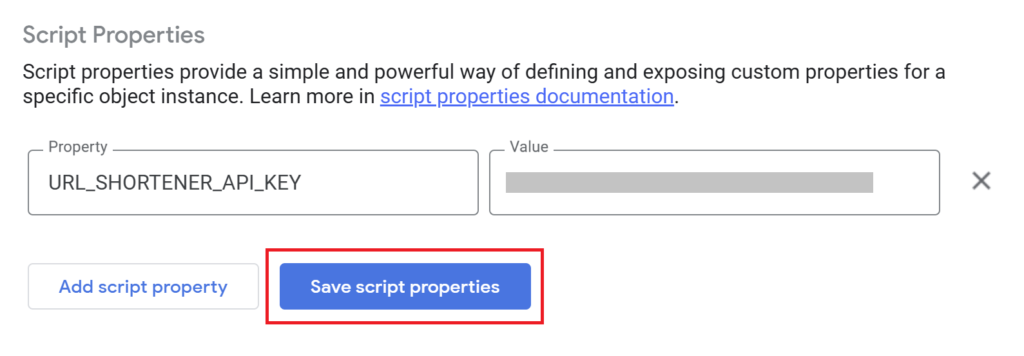
Once entered, click “Save Script Properties”.
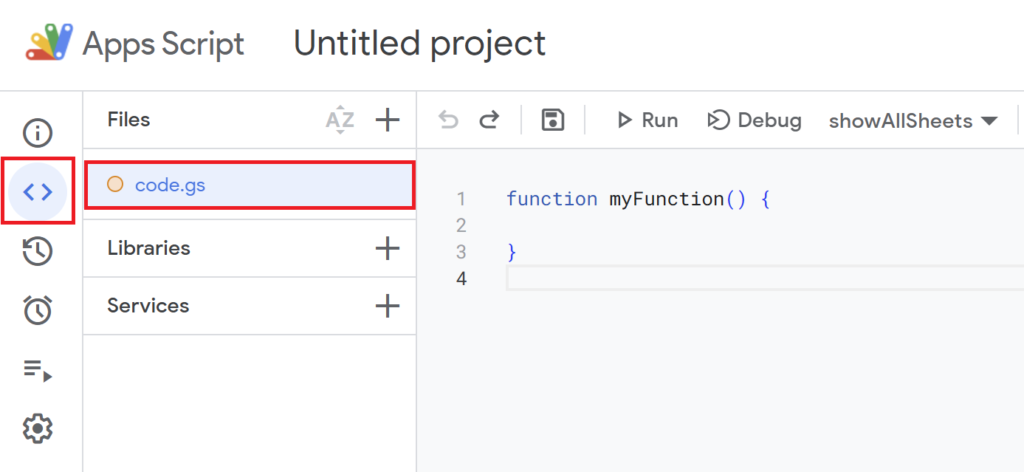
Since the default function myFunction() {}
is present, delete it and replace it with the script below.
function shortenUrls() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Shortened URLs"); // Specify the sheet name
if (!sheet) {
Logger.log("The specified sheet was not found.");
return;
}
var apiKey = PropertiesService.getScriptProperties().getProperty('URL_SHORTENER_API_KEY'); // Get API key from script properties
if (!apiKey) {
Logger.log("API key is not set.");
return;
}
var lastRow = sheet.getLastRow();
for (var i = 2; i <= lastRow; i++) { // Start from row 2 to skip headers
var longUrl = sheet.getRange(i, 1).getValue(); // Column A contains the original URLs
var existingShortUrl = sheet.getRange(i, 2).getValue(); // Column B stores shortened URLs
// Generate shortened URL only if column B is empty and column A has a value
if (longUrl && !existingShortUrl) {
var shortUrl = shortenUrlWithXgd(longUrl, apiKey);
sheet.getRange(i, 2).setValue(shortUrl); // Write the shortened URL in column B
}
}
}
function shortenUrlWithXgd(longUrl, apiKey) {
var url = "https://xgd.io/V1/shorten?url=" + encodeURIComponent(longUrl) + "&key=" + encodeURIComponent(apiKey);
var options = {
method: "GET",
muteHttpExceptions: true
};
var response = UrlFetchApp.fetch(url, options);
if (response.getResponseCode() !== 200) {
Logger.log("Error: " + response.getContentText());
throw new Error("Failed to shorten URL. Status code: " + response.getResponseCode());
}
var data = JSON.parse(response.getContentText());
Logger.log("Shortened URL: " + data.shorturl); // Log the shortened URL
return data.shorturl; // Return the shortened URL
}
Script Description
shortenUrls
Function-
This is the main process that retrieves the URLs from column A of the spreadsheet and outputs the shortened URLs in column B.
- Retrieve the Sheet
-
var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Shortened URLs"); // Specify the sheet name if (!sheet) { Logger.log("The specified sheet was not found."); return; }
- The script retrieves the sheet named “Shortened URLs” from the spreadsheet.
- Feel free to change the sheet name as needed.
- However, ensure the sheet name is correct; otherwise, the script will not function properly.
- Retrieve the API Key
-
var apiKey = PropertiesService.getScriptProperties().getProperty('URL_SHORTENER_API_KEY'); // Get API key from script properties if (!apiKey) { Logger.log("API key is not set."); return; }
- The script retrieves the API key stored in the Script Properties.
- If the API key is not set, an error message is logged, and the script terminates.
- Batch URL Processing
-
var lastRow = sheet.getLastRow(); for (var i = 2; i <= lastRow; i++) { // Start from row 2 to skip headers var longUrl = sheet.getRange(i, 1).getValue(); // Column A contains the original URLs var existingShortUrl = sheet.getRange(i, 2).getValue(); // Column B stores shortened URLs // Generate shortened URL only if column B is empty and column A has a value if (longUrl && !existingShortUrl) { var shortUrl = shortenUrlWithXgd(longUrl, apiKey); sheet.getRange(i, 2).setValue(shortUrl); // Write the shortened URL in column B } } }
- The script uses a loop (
for (var i = 2; i <= lastRow; i++) { ... }
) to process each row from the 2nd row to the last row. - Retrieves the URL from column A (
longUrl
). - Checks if a shortened URL already exists in column B (
existingShortUrl
). - If a URL is present in column A and column B is empty, it generates a shortened URL.
- The script uses a loop (
shortenUrlWithXgd
Function-
This function handles the actual URL shortening process using the X.gd API.
- APIリクエストの準備
-
var url = "https://xgd.io/V1/shorten?url=" + encodeURIComponent(longUrl) + "&key=" + encodeURIComponent(apiKey);
var url = "https://xgd.io/V1/shorten?url=" + encodeURIComponent(longUrl) + "&key=" + encodeURIComponent(apiKey);
specifies the endpoint for the X.gd API and appends the long URL and API key to the request.- Using
encodeURIComponent()
ensures the URL and API key are properly encoded, allowing the request to be sent securely.
- Sending the Request
-
var options = { method: "GET", muteHttpExceptions: true }; var response = UrlFetchApp.fetch(url, options);
- The script sends a GET request using
UrlFetchApp.fetch(url, options);
. - The
muteHttpExceptions: true
option allows the script to capture detailed error information in case the request fails.
- The script sends a GET request using
- Error Checking
-
if (response.getResponseCode() !== 200) { Logger.log("Error: " + response.getContentText()); throw new Error("Failed to shorten URL. Status code: " + response.getResponseCode()); }
- The script checks the request’s status code using
response.getResponseCode() !== 200
. - If the status code is not
200
, an error message is logged to provide details about the failure.
- The script checks the request’s status code using
- Retrieve and Return the Shortened URL
-
var data = JSON.parse(response.getContentText()); Logger.log("Shortened URL: " + data.shorturl); // Log the shortened URL return data.shorturl; // Return the shortened URL }
- The script parses the response content in JSON format and retrieves the shortened URL (
data.shorturl
). - This shortened URL is then returned and set in column B of the spreadsheet.
- The script parses the response content in JSON format and retrieves the shortened URL (
- Advantages of the Script
-
- Batch Processing
The script automatically shortens multiple URLs entered in column A, significantly reducing manual work. - Avoiding Duplicate Processing
Rows with existing shortened URLs in column B are skipped, preventing unnecessary API requests and saving resources. - Error Handling
If an API request fails, the script logs an error message, making it easy to identify and resolve issues.
- Batch Processing
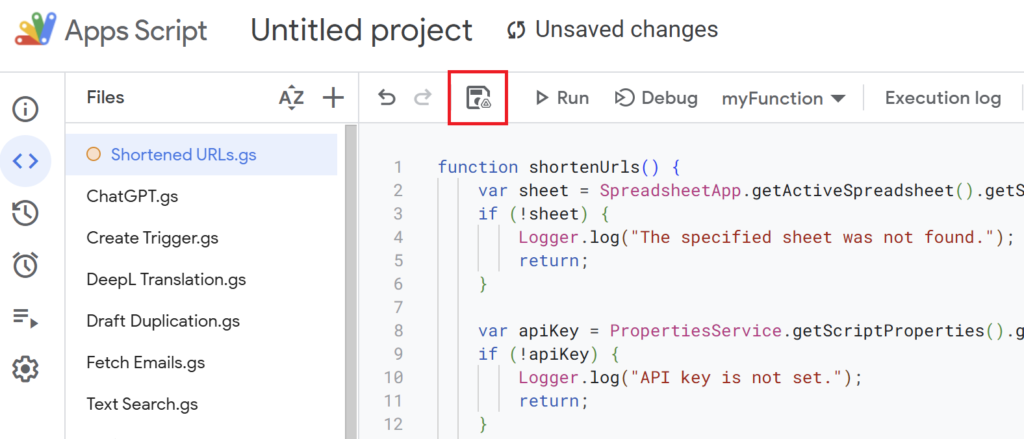
Once you’ve written the script, save it with a descriptive name.
(Example: “Shortened URLs”)
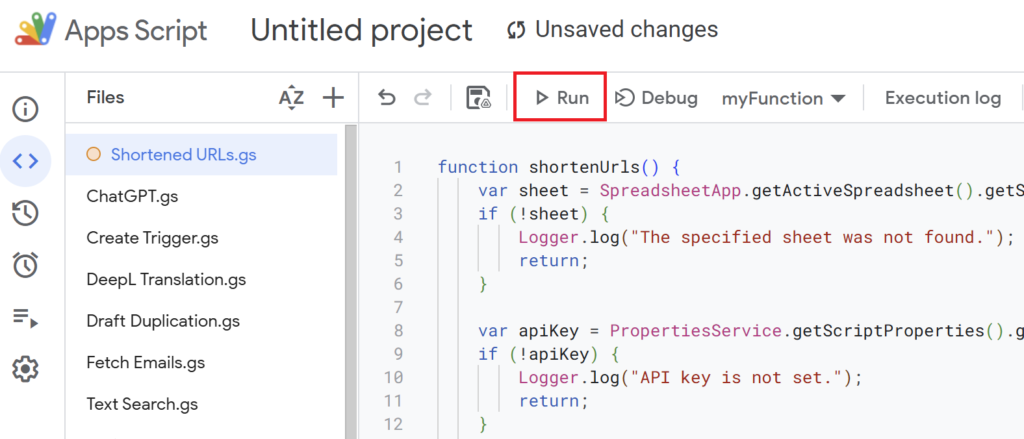
When you execute the script, shortened URLs will be generated and displayed in the spreadsheet.
If you’re running the script for the first time, you need to authorize it.
Therefore, press “Review Permissions.“
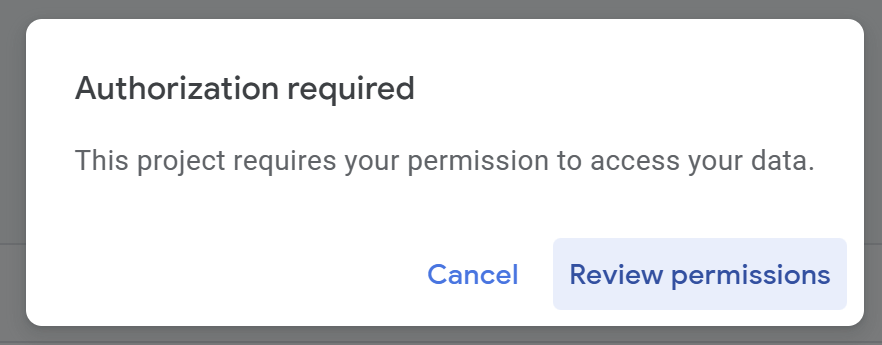
Detailed Authorization Steps
Press “Advanced.”
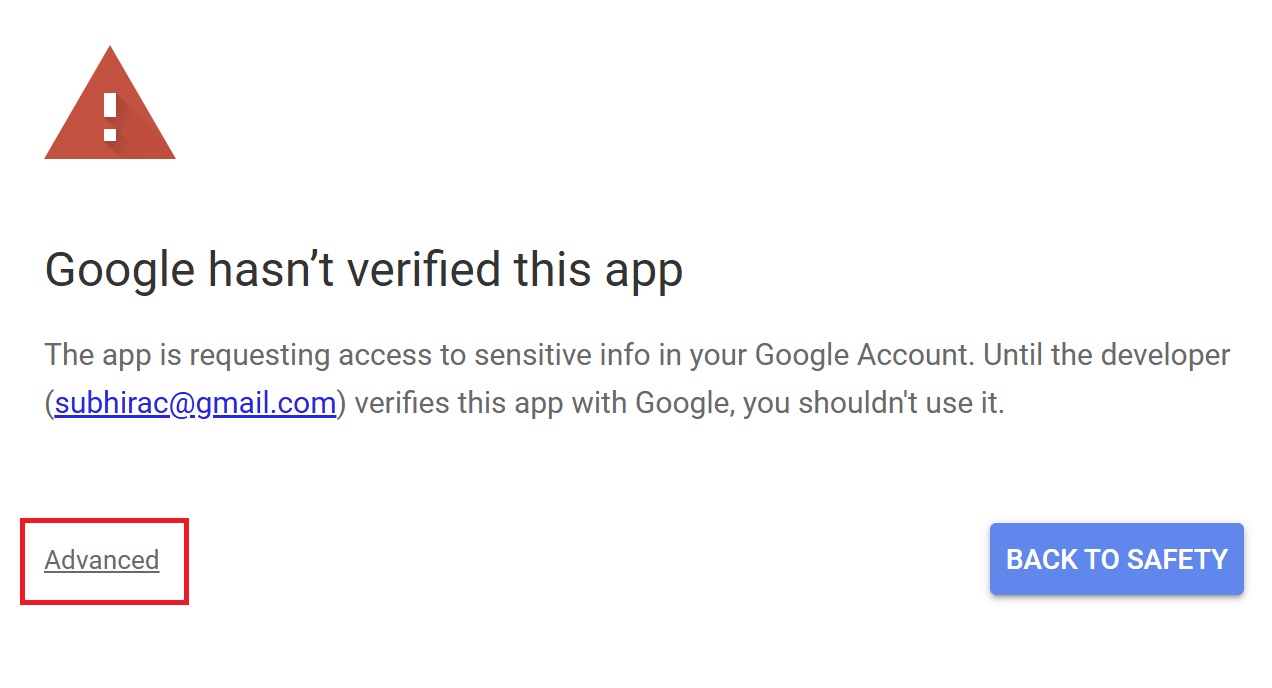
Press “Go to Untitled project (Unsafe).”
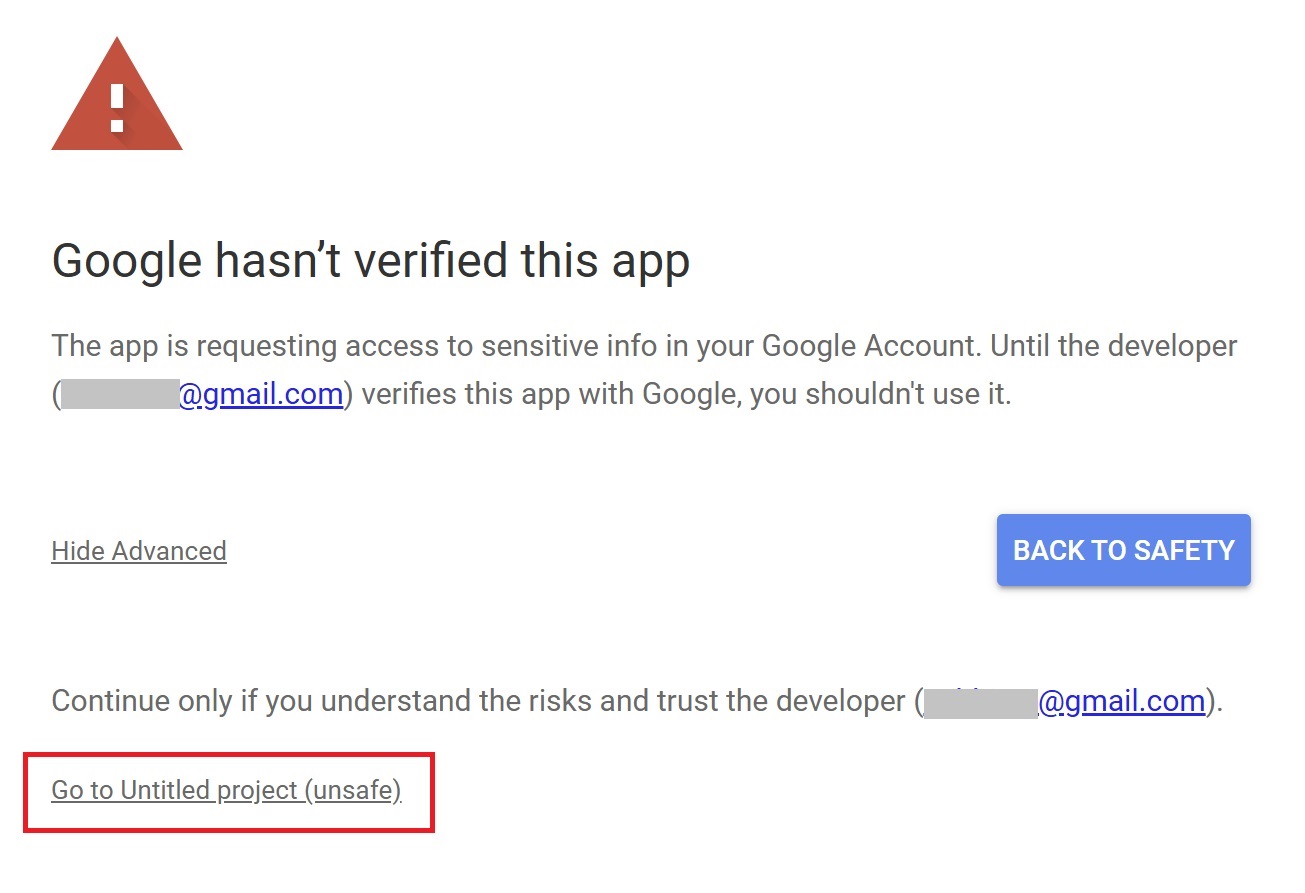
After that, press “Allow.”
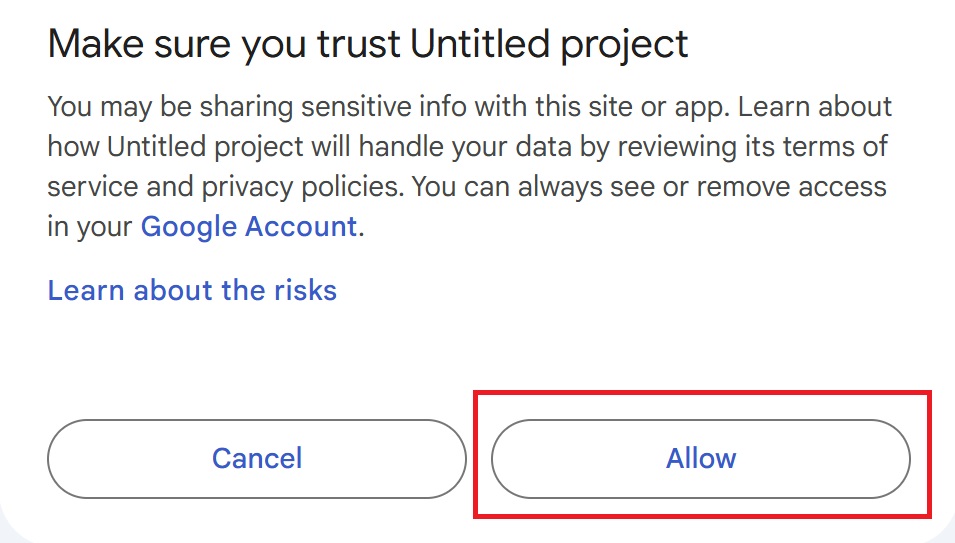
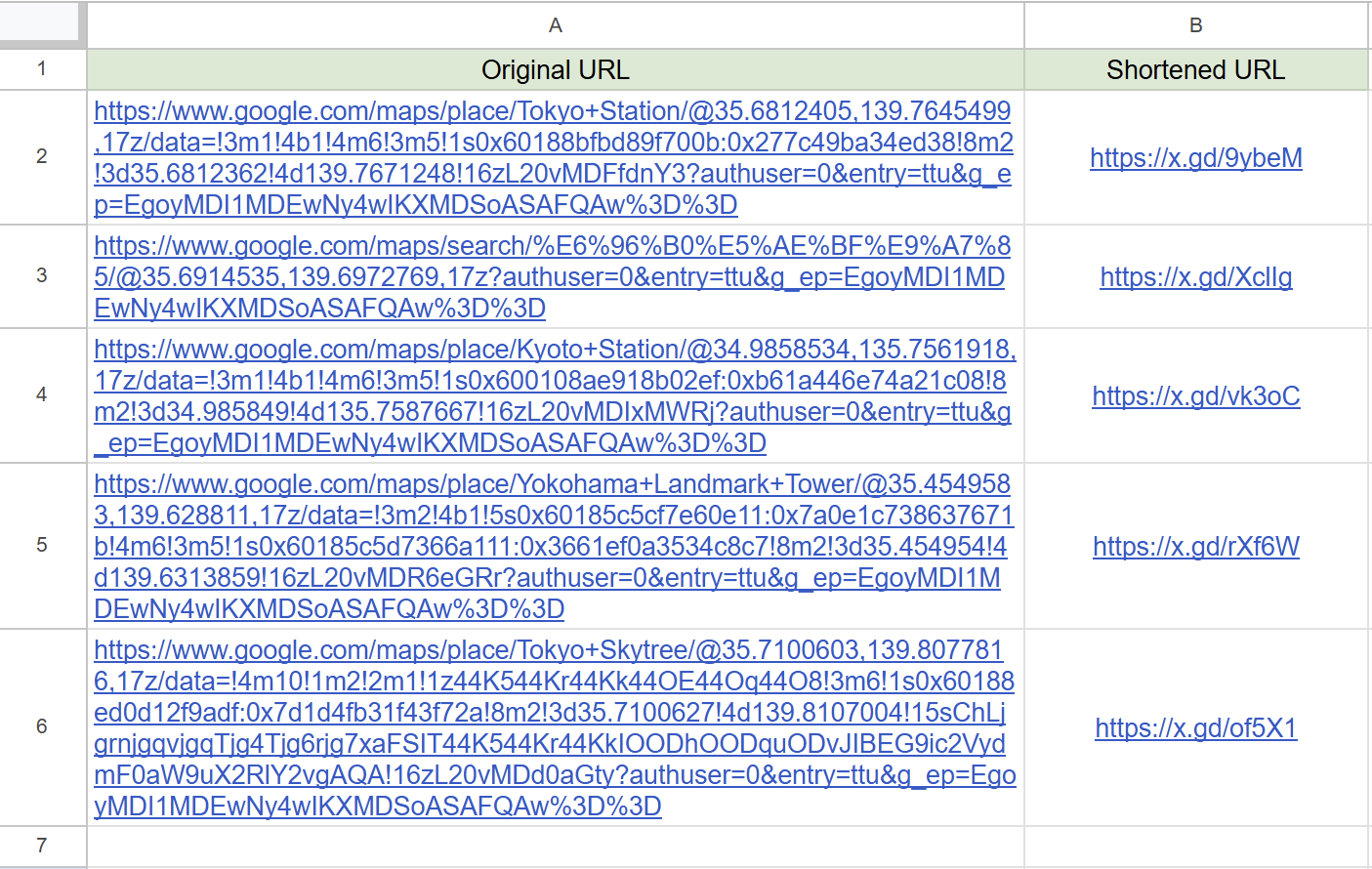
Based on the URLs entered in column A, shortened URLs are generated and displayed in column B.
To prevent overwriting, the script skips rows where a shortened URL already exists in column B, processing only the URLs that have not yet been shortened.
Conclusion
- If you want to shorten URLs one at a time, using a Chrome extension is convenient.
- For bulk URL shortening, Google Apps Script is the better option.
In this article, we introduced a method to bulk shorten multiple URLs using Google Sheets and Google Apps Script.
While a Chrome extension is great for shortening individual URLs, GAS allows you to efficiently process large numbers of URLs at once, making it ideal for handling bulk tasks.
We hope this helps you streamline your workflow and improve efficiency.
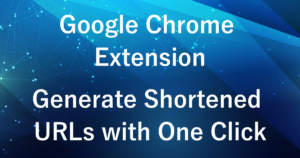
Our company offers support for improving work efficiency through the use of Google Apps Script.
If you need assistance with Google Apps Script customization or error resolution, please feel free to contact us.
We are fully committed to supporting your business improvements.
Contact us here
弊社では、Google Apps Script(GAS)を活用した業務効率化のサポートを提供しております。
GASのカスタマイズやエラー対応にお困りの際は、ぜひお気軽にご相談ください。
また、ITツールの導入支援やIT導入補助金の申請サポートも行っております。
貴方の業務改善を全力でサポートいたします。
Comments